随机森林模型 scala代码 RDD-based API roc曲线 混淆矩阵
时间: 2024-02-13 21:02:49 浏览: 23
好的,以下是使用RDD-based API实现随机森林模型的代码示例:
```scala
import org.apache.spark.SparkConf
import org.apache.spark.SparkContext
import org.apache.spark.mllib.tree.RandomForest
import org.apache.spark.mllib.tree.model.RandomForestModel
import org.apache.spark.mllib.util.MLUtils
import org.apache.spark.rdd.RDD
object RandomForestModel {
def main(args: Array[String]): Unit = {
val conf = new SparkConf().setAppName("RandomForestModel").setMaster("local[*]")
val sc = new SparkContext(conf)
// 读取数据
val data = MLUtils.loadLibSVMFile(sc, "path/to/data.txt")
// 划分数据集
val Array(trainingData, testData) = data.randomSplit(Array(0.7, 0.3))
// 定义随机森林模型参数
val numClasses = 2
val categoricalFeaturesInfo = Map[Int, Int]()
val numTrees = 10
val featureSubsetStrategy = "auto"
val impurity = "gini"
val maxDepth = 4
val maxBins = 32
// 训练模型
val model = RandomForest.trainClassifier(trainingData, numClasses, categoricalFeaturesInfo, numTrees, featureSubsetStrategy, impurity, maxDepth, maxBins)
// 预测
val predictionsAndLabels: RDD[(Double, Double)] = testData.map { point =>
val prediction = model.predict(point.features)
(prediction, point.label)
}
// 评估模型
val testErr = 1.0 * predictionsAndLabels.filter(r => r._1 != r._2).count() / testData.count()
println(s"Test Error = $testErr")
// 输出ROC曲线
val metrics = new BinaryClassificationMetrics(predictionsAndLabels)
val roc = metrics.roc()
roc.foreach { case (tpr, fpr, threshold) =>
println(s"$fpr, $tpr")
}
// 输出混淆矩阵
val labels = predictionsAndLabels.map(_._2).distinct().collect()
val confusionMatrix = predictionsAndLabels.filter { case (predicted, actual) => predicted == actual }
.countByValue(labels)
println(s"Confusion matrix: ${confusionMatrix.mkString(", ")}")
}
}
```
以上代码中,我们使用了Spark MLlib提供的随机森林分类器(RandomForest)来训练模型,并对测试集进行预测和评估。同时,我们还使用了评估指标中的ROC曲线和混淆矩阵来评价模型的性能,分别使用了`BinaryClassificationMetrics`类中的`roc()`和`countByValue()`方法来输出结果。
相关推荐
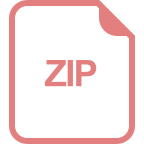
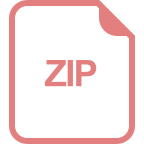
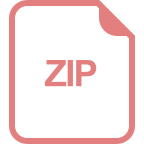














