SDL打印Hello World
时间: 2024-09-14 12:09:04 浏览: 38
在SDL(Simple DirectMedia Layer)中,打印"Hello World"主要是通过绘制文本到屏幕上来完成的。以下是简单的步骤和代码示例:
1. 首先,需要初始化SDL库和窗口。这包括创建一个窗口,设定大小和标题:
```c++
#include "SDL.h"
int main(int argc, char* args[]) {
if (SDL_Init(SDL_INIT_VIDEO) < 0) {
printf("SDL could not initialize! Error: %s\n", SDL_GetError());
return 1;
}
SDL_Window* window = SDL_CreateWindow("Hello World!", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 300, 200, SDL_WINDOW_SHOWN);
if (window == NULL) {
printf("Window could not be created! Error: %s\n", SDL_GetError());
SDL_Quit();
return 1;
}
}
```
2. 接下来,我们需要渲染字体。这通常通过加载一个字体文件(如.TTF格式)来实现,例如:
```c++
TTF_Font* font = TTF_OpenFont("arial.ttf", 32);
if (!font) {
printf("Could not load font! Error: %s\n", TTF_GetError());
SDL_DestroyWindow(window);
SDL_Quit();
return 1;
}
```
3. 然后,在合适的位置创建一个表面(Surface),并在上面绘制文字:
```c++
SDL_Surface* messageSurface = TTF_RenderText_Solid(font, "Hello World!", SDL_Color{255, 255, 255, 255});
if (messageSurface == NULL) {
printf("Could not render text! Error: %s\n", TTF_GetError());
SDL_DestroyFont(font);
SDL_DestroyWindow(window);
SDL_Quit();
return 1;
}
// Blit the surface to the screen
SDL_Rect rect;
rect.x = 50; // x position
rect.y = 50; // y position
SDL_BlitSurface(messageSurface, NULL, window, &rect);
```
4. 最后,别忘了刷新窗口和运行主循环:
```c++
while (true) {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
break;
}
}
SDL_UpdateWindowSurface(window); // 更新窗口显示
SDL_Delay(1000 / 60); // 暂停一下防止过快
}
```
记得在退出程序时释放所有资源:
```c++
SDL_FreeSurface(messageSurface);
TTF_CloseFont(font);
SDL_DestroyWindow(window);
SDL_Quit();
```
阅读全文
相关推荐
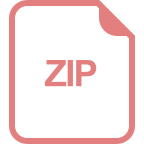
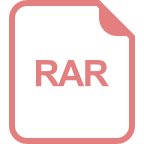
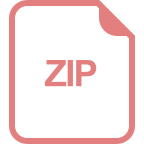
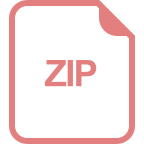
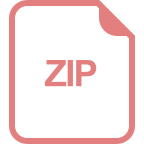
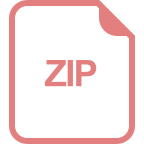
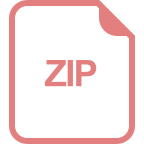
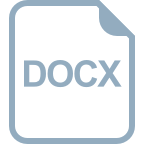
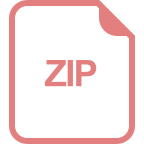
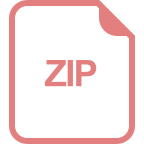
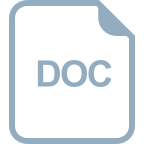
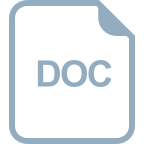
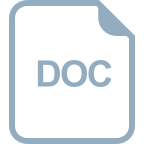





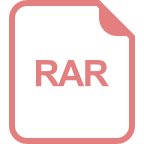