jsonobject转list排序
时间: 2023-07-22 21:04:39 浏览: 68
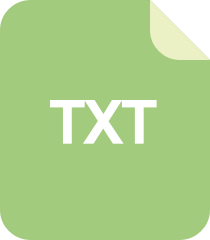
JsonObject转换Date日期

首先,你需要将JSONObject对象转换成List对象,可以通过遍历JSONObject中的键值对来实现:
```java
JSONObject jsonObject = new JSONObject("{\"name\":\"Alice\",\"age\":20,\"gender\":\"female\"}");
List<Map.Entry<String, Object>> list = new ArrayList<>();
for (Map.Entry<String, Object> entry : jsonObject.toMap().entrySet()) {
list.add(entry);
}
```
然后,你可以通过Collections.sort()方法来对List进行排序,可以根据值的大小或键的字母顺序排序:
```java
// 根据键的字母顺序排序
Collections.sort(list, new Comparator<Map.Entry<String, Object>>() {
public int compare(Map.Entry<String, Object> o1, Map.Entry<String, Object> o2) {
return o1.getKey().compareTo(o2.getKey());
}
});
// 根据值的大小排序
Collections.sort(list, new Comparator<Map.Entry<String, Object>>() {
public int compare(Map.Entry<String, Object> o1, Map.Entry<String, Object> o2) {
if (o1.getValue() instanceof Number && o2.getValue() instanceof Number) {
return Integer.compare(((Number) o1.getValue()).intValue(), ((Number) o2.getValue()).intValue());
} else {
return o1.getValue().toString().compareTo(o2.getValue().toString());
}
}
});
```
最后,你可以将排序后的List转换回JSONObject对象:
```java
JSONObject sortedJsonObject = new JSONObject();
for (Map.Entry<String, Object> entry : list) {
sortedJsonObject.put(entry.getKey(), entry.getValue());
}
```
阅读全文
相关推荐
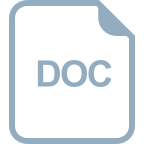
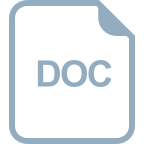












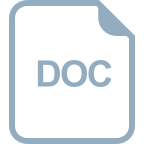
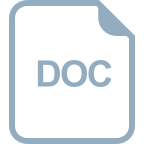
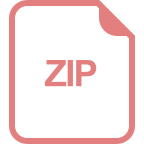