Java 手写线程池,并封装异步执行器
时间: 2023-07-06 17:35:01 浏览: 110
好的,我可以为您手写一个线程池,并封装异步执行器。以下是示例代码:
```java
import java.util.concurrent.*;
public class ThreadPool {
private final BlockingQueue<Runnable> taskQueue;
private final Thread[] threads;
public ThreadPool(int numThreads, int queueSize) {
taskQueue = new ArrayBlockingQueue<>(queueSize);
threads = new Thread[numThreads];
for (int i = 0; i < numThreads; i++) {
threads[i] = new Thread(() -> {
while (true) {
try {
Runnable task = taskQueue.take();
task.run();
} catch (InterruptedException e) {
break;
}
}
});
threads[i].start();
}
}
public void execute(Runnable task) throws InterruptedException {
taskQueue.put(task);
}
public void shutdown() throws InterruptedException {
for (Thread thread : threads) {
thread.interrupt();
}
for (Thread thread : threads) {
thread.join();
}
}
}
```
使用示例:
```java
ThreadPool threadPool = new ThreadPool(10, 100);
for (int i = 0; i < 50; i++) {
final int j = i;
threadPool.execute(() -> {
System.out.println("Task " + j + " executed by " + Thread.currentThread().getName());
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
}
threadPool.shutdown();
```
这个线程池使用了Java标准库中的BlockingQueue和Thread,允许您提交任务并异步执行。您可以根据需要调整线程池的大小和任务队列的大小。注意在使用完线程池后需要调用shutdown()方法关闭线程池。接下来,我们可以将线程池封装为异步执行器:
```java
import java.util.concurrent.*;
public class AsyncExecutor {
private final ThreadPool threadPool;
public AsyncExecutor(int numThreads, int queueSize) {
threadPool = new ThreadPool(numThreads, queueSize);
}
public void execute(Runnable task) throws InterruptedException {
threadPool.execute(task);
}
public void shutdown() throws InterruptedException {
threadPool.shutdown();
}
}
```
这个异步执行器使用我们手写的线程池,允许您提交任务并异步执行。您可以根据需要调整线程池的大小和任务队列的大小。注意在使用完异步执行器后需要调用shutdown()方法关闭线程池。
阅读全文
相关推荐
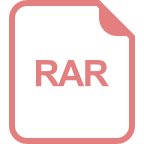
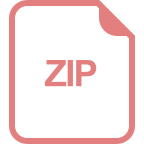
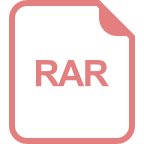
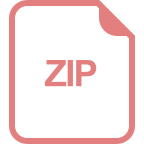
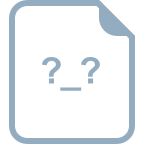
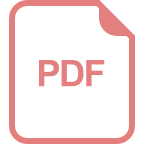
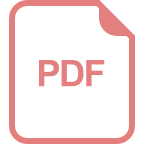
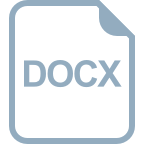
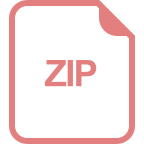
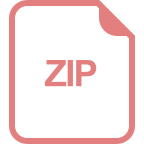
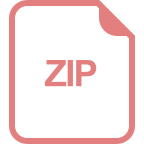
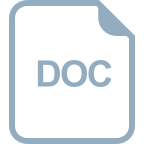
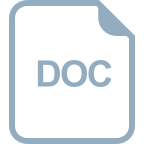
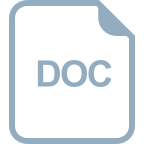
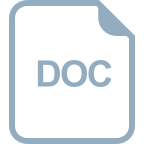
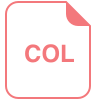