QGraphicsScene查看场景中的图元数量
时间: 2024-02-22 09:56:05 浏览: 32
您可以使用QGraphicsScene的items()方法来获取场景中所有图元的列表,然后使用count()方法来获取图元数量。示例代码如下:
```
QGraphicsScene* scene = new QGraphicsScene();
// 添加图元到场景中
QGraphicsEllipseItem* ellipse = scene->addEllipse(0, 0, 100, 100);
QGraphicsRectItem* rect = scene->addRect(0, 0, 100, 100);
// 获取场景中所有图元的列表
QList<QGraphicsItem*> items = scene->items();
// 获取图元数量
int count = items.count();
```
在上面的示例中,我们创建了一个QGraphicsScene并向其中添加了两个图元。然后,我们使用items()方法获取场景中所有图元的列表,并使用count()方法获取图元数量。
相关问题
遍历qgraphicsscene中的图元
在QGraphicsScene中,可以通过使用QGraphicsScene::items()函数来遍历图元。
QGraphicsScene::items()函数返回一个QList<QGraphicsItem*>对象,包含了场景中所有的图元。我们可以使用迭代器来遍历这个列表,以获取每个图元的详细信息。
例如,下面是一个用于遍历并打印场景中所有图元信息的示例代码:
```cpp
QGraphicsScene scene;
// 在场景中添加一些图元...
QList<QGraphicsItem*> items = scene.items();
for (QGraphicsItem* item : items) {
// 打印图元的类型
qDebug() << "图元类型:" << item->type();
// 打印图元的位置和大小
qDebug() << "图元位置:" << item->pos();
qDebug() << "图元大小:" << item->boundingRect().size();
// 打印图元的其他属性
// ...
// 你还可以对图元进行其他操作,如修改属性、删除等
// ...
}
```
在上面的示例代码中,我们先通过QGraphicsScene::items()获取场景中的所有图元,并将它们保存在一个QList<QGraphicsItem*>对象中。然后,我们使用for循环遍历该列表,对每个图元进行操作。
你可以根据具体需求,进一步扩展该代码来实现不同的功能,如根据图元类型执行不同的操作,或根据图元属性进行筛选等等。希望对你有所帮助!
pyqt5 qgraphicsscene 多场景切换
在PyQt5中,可以通过QGraphicsScene来创建多个场景,并在需要的时候进行场景切换。
首先,我们需要创建多个QGraphicsScene对象,并将它们分别添加到QGraphicsView中,以便能够显示出来。可以使用QStackedLayout来管理QGraphicsView,以实现场景的切换。
接下来,需要为每个场景定义相应的功能和交互,例如添加图形项、处理鼠标事件等。可以通过继承QGraphicsScene类来创建自定义场景,然后重写需要的方法。
对于场景切换,可以使用QPushButton等按钮控件来触发切换动作。在按钮的点击事件中,调用QStackedLayout的setCurrentIndex方法来切换场景。
另外,还可以添加过渡动画来实现平滑的场景切换效果。可以使用QPropertyAnimation类来实现图形项的动画效果,例如平移、旋转等。通过在场景切换时添加动画效果,可以增强用户的交互体验。
总结起来,实现PyQt5中QGraphicsScene的多场景切换可以通过以下步骤:
1. 创建多个QGraphicsScene对象并添加到QGraphicsView中。
2. 为每个场景定义相应的功能和交互。
3. 使用QStackedLayout管理QGraphicsView,以实现场景的切换。
4. 使用控件触发场景切换,并调用setCurrentIndex方法来实现切换。
5. 可选:添加过渡动画来实现平滑的场景切换效果。
相关推荐
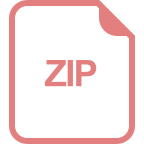












