/dev/ttyUSB如何调用,需要安装驱动吗
时间: 2023-12-02 08:02:30 浏览: 96
/dev/ttyUSB是Linux系统中用于连接USB设备的串口设备文件,它不需要安装驱动。当你连接一个USB串口设备(如Arduino、串口转USB模块等)时,系统会自动识别设备,并在/dev目录下创建对应的设备文件,比如/dev/ttyUSB0。你可以通过在终端中输入以下命令来查看设备是否已经识别:
```
ls /dev/ttyUSB*
```
如果该设备已经被识别,则会显示出对应的设备文件。接下来,你可以使用串口通信程序(如minicom)来对设备进行通信。
相关问题
/dev/ttyUSB0
/dev/ttyUSB0是Linux系统中用于连接USB设备的串口设备文件。它是通过udev规则自动创建的,可以用于与USB设备进行通信。
要查看/dev/ttyUSB0设备的信息,可以使用以下命令:
```shell
ls -l /dev/ttyUSB0
```
该命令将显示有关设备文件的详细信息,包括文件权限、所有者、组、大小和创建日期等。
如果要查看所有以/dev/ttyUSB开头的设备文件,可以使用以下命令:
```shell
ls -l /dev/ttyUSB*
```
该命令将显示所有以/dev/ttyUSB开头的设备文件的详细信息。
请注意,要访问/dev/ttyUSB0设备文件,您可能需要具有适当的权限。通常,您需要将用户添加到dialout组中,以便具有对串口设备的读写权限。您可以使用以下命令将用户添加到dialout组:
```shell
sudo usermod -aG dialout <用户名>
```
/dev/ttyUSB
/dev/ttyUSB是Linux系统中的一个设备文件,用于表示USB串口设备。在Linux系统中,串口设备通常以/dev/ttyUSB0、/dev/ttyUSB1等形式命名。这些设备文件可以用于与外部设备进行串口通信,例如与Arduino、传感器等进行数据交互。
/dev/ttyUSB是一种特殊的设备文件,它提供了对USB串口设备的访问权限。通过打开/dev/ttyUSB文件,可以向串口设备发送数据或从串口设备接收数据。在Linux系统中,可以使用各种编程语言(如C、C++、Python等)来编写程序,通过读写/dev/ttyUSB文件来实现与USB串口设备的通信。
相关推荐
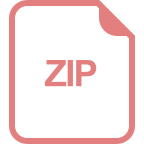
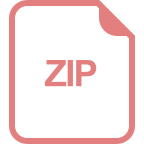












