用c语言解决这个问题:描述: Erik is a knight who can save the princess out of the maze, so Erik walked into the maze, a 4-direction maze (left, up, right, down), and found the princess. Now Erik wants to walk to the exit of the maze. Luckily Erik has a map of this maze. The maze consists of n*m grids, with each grid being one of the following types: T: representing Erik can walk to this grid. F: representing Erik cannot walk to this grid, because of danger. B: representing Erik's beginning position. E: representing the exit of the maze. If the grid's type is T and Erik walks from this gird to another, then the gird's type will change to F. Also, Erik cannot pass B and E twice or more. Now, Erik wants to know how many different ways he can get out of the maze. 输入:The first line contains two integers n and m (1<=n<=6,1<=m<=6). There are n more lines in the case, each of which includes m characters. Each character represents the type of a grid, that is, T, F, B or E. 输出:Output a number representing the number of different ways in which Erik can get out of the maze.
时间: 2024-02-19 18:59:22 浏览: 19
这个问题可以使用深度优先搜索(DFS)算法来解决。具体步骤如下:
1. 读入输入数据,将起点和终点的位置记录下来。
2. 定义一个二维数组 maze 存储迷宫的地图,使用一个一维数组 visited 记录每个位置是否已经被访问过。
3. 定义一个计数器 count,初始值为 0。
4. 定义一个 DFS 函数,输入参数为当前位置的行、列坐标和已经走过的步数 step。
5. 如果当前位置已经是终点位置,则将 count 加 1。
6. 否则,遍历当前位置的四个邻居位置(上下左右),如果该邻居位置是合法的、未被访问过且可以到达(即该位置是 T),则将该邻居位置的 visited 标记为已访问,并将该位置的类型改为 F,然后递归调用 DFS 函数,更新当前位置为邻居位置,步数加一,并将新的位置和步数作为参数传给 DFS 函数。
7. 在 DFS 函数结束后,将当前位置的 visited 标记为未访问过,将该位置的类型改回 T(回溯过程)。
8. 最后输出 count 的值即可。
以下是完整的 C 语言代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_N 6
#define MAX_M 6
int n, m, start_row, start_col, end_row, end_col;
char maze[MAX_N][MAX_M + 1];
int visited[MAX_N][MAX_M];
int count = 0;
void dfs(int row, int col, int step)
{
if (row == end_row && col == end_col) {
count++;
return;
}
visited[row][col] = 1;
int dr[4] = {-1, 0, 1, 0};
int dc[4] = {0, 1, 0, -1};
for (int i = 0; i < 4; i++) {
int new_row = row + dr[i];
int new_col = col + dc[i];
if (new_row >= 0 && new_row < n && new_col >= 0 && new_col < m
&& !visited[new_row][new_col] && maze[new_row][new_col] == 'T') {
maze[new_row][new_col] = 'F';
dfs(new_row, new_col, step + 1);
maze[new_row][new_col] = 'T';
}
}
visited[row][col] = 0;
}
int main()
{
scanf("%d%d", &n, &m);
for (int i = 0; i < n; i++) {
scanf("%s", maze[i]);
for (int j = 0; j < m; j++) {
if (maze[i][j] == 'B') {
start_row = i;
start_col = j;
} else if (maze[i][j] == 'E') {
end_row = i;
end_col = j;
}
}
}
memset(visited, 0, sizeof(visited));
dfs(start_row, start_col, 0);
printf("%d\n", count);
return 0;
}
```
注意,本题中 n 和 m 的范围比较小,因此可以使用递归的深度优先搜索算法。如果 n 和 m 的范围较大,可能需要使用迭代的深度优先搜索算法或广度优先搜索算法来解决。
相关推荐
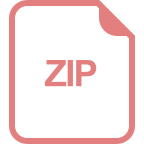
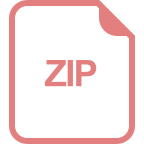
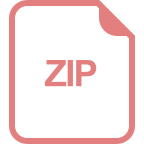














