使用Vue编写一个购物车
时间: 2023-09-17 19:08:52 浏览: 50
好的,下面是一个简单的Vue购物车示例:
HTML模板:
```html
<div id="app">
<h2>商品列表</h2>
<ul>
<li v-for="(item, index) in goods" :key="index">
{{ item.name }} - ¥{{ item.price }}
<button @click="addToCart(item)">加入购物车</button>
</li>
</ul>
<h2>购物车</h2>
<ul>
<li v-for="(item, index) in cart" :key="index">
{{ item.name }} - ¥{{ item.price }} x {{ item.quantity }}
<button @click="removeFromCart(item)">删除</button>
</li>
</ul>
<div>
总价:¥{{ totalPrice }}
</div>
</div>
```
Vue实例:
```javascript
new Vue({
el: '#app',
data: {
goods: [
{ name: '商品1', price: 10 },
{ name: '商品2', price: 20 },
{ name: '商品3', price: 30 },
],
cart: [],
},
computed: {
totalPrice() {
return this.cart.reduce((total, item) => total + item.price * item.quantity, 0);
},
},
methods: {
addToCart(item) {
const index = this.cart.findIndex(i => i.name === item.name);
if (index >= 0) {
this.cart[index].quantity += 1;
} else {
this.cart.push({
name: item.name,
price: item.price,
quantity: 1,
});
}
},
removeFromCart(item) {
const index = this.cart.findIndex(i => i.name === item.name);
if (index >= 0) {
if (this.cart[index].quantity > 1) {
this.cart[index].quantity -= 1;
} else {
this.cart.splice(index, 1);
}
}
},
},
});
```
这个示例中,我们使用了Vue的数据绑定、计算属性和方法等功能,实现了一个简单的购物车功能。具体来说,我们在Vue实例中定义了`goods`和`cart`两个数组,分别表示商品列表和购物车列表。在模板中,我们使用了`v-for`指令循环展示商品列表和购物车列表,并为加入购物车按钮和删除按钮添加了点击事件。在Vue实例中,我们定义了两个方法`addToCart`和`removeFromCart`,分别用于将商品添加到购物车和从购物车中删除商品,同时在计算属性`totalPrice`中计算出购物车的总价。
相关推荐
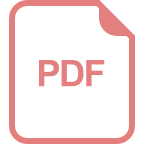
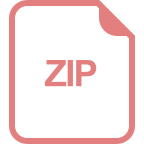
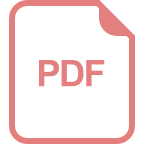














