编写五子棋的完整python代码_python实现五子棋程序
时间: 2024-03-02 12:24:28 浏览: 23
以下是一个简单的 Python 五子棋游戏代码,使用了 Pygame 模块。
```python
import pygame
import sys
import numpy as np
# 初始化 Pygame
pygame.init()
# 设置窗口尺寸
WIDTH = 640
HEIGHT = 640
# 设置棋盘尺寸
BOARD_SIZE = 15
CELL_SIZE = int(WIDTH / BOARD_SIZE)
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
# 创建窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('五子棋')
# 创建棋盘
board = np.zeros((BOARD_SIZE, BOARD_SIZE))
# 判断是否有五子连珠
def is_win(player):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == player:
# 判断横向是否有五个相同棋子
if j + 4 < BOARD_SIZE and board[i][j+1] == player and board[i][j+2] == player and board[i][j+3] == player and board[i][j+4] == player:
return True
# 判断纵向是否有五个相同棋子
if i + 4 < BOARD_SIZE and board[i+1][j] == player and board[i+2][j] == player and board[i+3][j] == player and board[i+4][j] == player:
return True
# 判断左上到右下是否有五个相同棋子
if i + 4 < BOARD_SIZE and j + 4 < BOARD_SIZE and board[i+1][j+1] == player and board[i+2][j+2] == player and board[i+3][j+3] == player and board[i+4][j+4] == player:
return True
# 判断右上到左下是否有五个相同棋子
if i + 4 < BOARD_SIZE and j - 4 >= 0 and board[i+1][j-1] == player and board[i+2][j-2] == player and board[i+3][j-3] == player and board[i+4][j-4] == player:
return True
return False
# 画棋盘
def draw_board():
# 填充背景色
screen.fill(GRAY)
# 画横线
for i in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (0, i * CELL_SIZE), (WIDTH, i * CELL_SIZE), 2)
# 画竖线
for j in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (j * CELL_SIZE, 0), (j * CELL_SIZE, HEIGHT), 2)
# 画棋子
def draw_piece(i, j, player):
center = ((j * CELL_SIZE) + CELL_SIZE // 2, (i * CELL_SIZE) + CELL_SIZE // 2)
if player == 1:
pygame.draw.circle(screen, BLACK, center, CELL_SIZE // 2 - 2)
else:
pygame.draw.circle(screen, WHITE, center, CELL_SIZE // 2 - 2)
# 游戏主循环
def main():
# 当前玩家
current_player = 1
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = pygame.mouse.get_pos()
i = y // CELL_SIZE
j = x // CELL_SIZE
if board[i][j] == 0:
board[i][j] = current_player
draw_piece(i, j, current_player)
if is_win(current_player):
print('玩家 %d 获胜!' % current_player)
pygame.quit()
sys.exit()
current_player = 3 - current_player
# 画棋盘和棋子
draw_board()
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] != 0:
draw_piece(i, j, board[i][j])
# 更新屏幕
pygame.display.update()
# 运行游戏
if __name__ == '__main__':
main()
```
这是一个简单的五子棋游戏,只实现了基本的操作和判断胜负。你可以根据自己的需求进行修改和扩展。
相关推荐
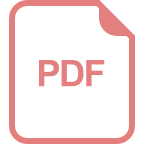
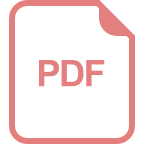







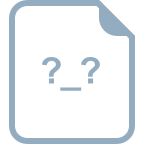
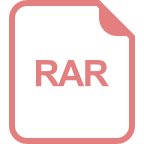