利用可扩展 hash 方法对以下记录进行 hash 存储: 3, 5, 7, 12, 16 设 hash 函数 h(x)= x mod 8,其中散列函数 h(k)是一个 b(足够大)位二进制序列,序列的前d位用作索引,来区分每个元素属于哪个桶。 现要求每个桶至多包含2个元素,以上元素按从左往右的顺序依次添加。开始时只使用 序列的前1位作索引(即d=1),当桶满时进行分裂,d相应增大。请画出添加完以上 所有元素后,最终的索引结构
时间: 2024-03-26 08:42:16 浏览: 42
以下是添加完以上所有元素后,最终的索引结构:
```
d=1:
0:
1: 3, 5
2:
3: 7
4:
5:
6: 12, 16
7:
d=2:
00:
01: 3, 5
10:
11: 7
100:
101:
110: 12, 16
111:
```
初始时,因为只使用序列的前1位作索引,所以所有元素都被散列到了同一个桶中。当桶满时,对应的索引位置增加一位(d=2),并将桶中的元素分到新的桶中。这样,每个桶至多包含2个元素。
相关问题
编写代码:使用双散列法查找节点插入位置,第一次散列函数为Hash(x)=(3*x)%11,第二次散列函数为Hash(x)=(7*x)%10+1
下面是使用双散列法查找节点插入位置的示例代码,其中第一次散列函数为`Hash(x) = (3*x) % 11`,第二次散列函数为`Hash(x) = (7*x) % 10 + 1`:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 哈希表中的节点结构体
struct Node {
int key;
int value;
};
// 哈希表结构体
struct HashTable {
vector<Node*> buckets;
int size;
HashTable(int size): size(size) {
buckets.resize(size);
}
};
// 第一次散列函数
int hash1(int x) {
return (3*x) % 11;
}
// 第二次散列函数
int hash2(int x) {
return (7*x) % 10 + 1;
}
// 双散列法查找节点插入位置
int doubleHashInsert(HashTable *ht, int key) {
int index = hash1(key); // 计算初始位置
int step = hash2(key); // 计算步长
int count = 0; // 记录插入次数
while (ht->buckets[index] != nullptr && ht->buckets[index]->key != key) { // 如果该位置已被占用且不是要插入的节点
index = (index + step) % ht->size; // 计算下一个位置
count++;
if (count == ht->size) { // 如果已经插入了哈希表大小次,说明哈希表已满
return -1;
}
}
return index; // 返回插入位置
}
int main() {
HashTable ht(11);
Node n1 = {1, 10};
Node n2 = {2, 20};
Node n3 = {3, 30};
Node n4 = {4, 40};
Node n5 = {5, 50};
ht.buckets[doubleHashInsert(&ht, n1.key)] = &n1;
ht.buckets[doubleHashInsert(&ht, n2.key)] = &n2;
ht.buckets[doubleHashInsert(&ht, n3.key)] = &n3;
ht.buckets[doubleHashInsert(&ht, n4.key)] = &n4;
ht.buckets[doubleHashInsert(&ht, n5.key)] = &n5;
for (int i = 0; i < ht.size; i++) {
if (ht.buckets[i] != nullptr) {
cout << "key: " << ht.buckets[i]->key << ", value: " << ht.buckets[i]->value << endl;
}
}
return 0;
}
```
在上述代码中,我们首先定义了`Node`和`HashTable`结构体。`HashTable`结构体中使用了`vector`来存储哈希表中的节点,同时定义了一个大小为`size`的哈希表。接下来,我们定义了第一次散列函数`hash1(x) = (3*x) % 11`和第二次散列函数`hash2(x) = (7*x) % 10 + 1`。最后,我们使用`doubleHashInsert()`函数向哈希表中插入了5个节点,并输出了哈希表中的所有节点。
需要注意的是,在双散列法中,第二次散列函数的返回值不能为0,因为如果步长为0,那么每次计算下一个位置时都会停留在同一个位置,无法插入哈希表中。因此,在上述代码中,我们将第二次散列函数的返回值加1,确保步长不为0。
编写代码实现使用time或datetime库将时间戳附加到创建或挖掘的每个块。hahshlib将用于散列块,在我们散列之前将使用JSON对块进行编码。 5.任务3:构建区块链架构 要开始构建PoW区块链,请创建一个Biockchain类。The_init方法将由一个名为chain的变量组成,用于存储区块链中所有区块的列表。 生成的区块链结构如下所示 i index: 0,#创世区块时间步长:当前时间,data:“Hello World!”,证明:0, previous_hash:“o”}->hash() -> 3s2351h i 指数:1 时间步长:当前时间,data:“交易A”,证明:24912, previous_hash: 3 s2351h}->hash() -> 8fv743413 i 指数:2 时间步长:当前时间,data:“交易B”,证明:235724, previous_hash: 8 fv743413 大数据-信息安全 Lab:用Python构建区块链 3 }->hash()->41j566d098 Task3.1:编写一个块生成函数,在类的实例化时创建Genesis块。 Index:索引键将存储区块链的长度。它由initmethod中的chain变量表示。
好的,您的问题是如何编写一个块生成函数,以及如何在类的实例化时创建Genesis块。
首先,我们需要导入需要使用的库:
```python
import hashlib
import json
import time
```
然后,我们可以定义一个块生成函数:
```python
def generate_block(index, timestamp, data, proof, previous_hash):
"""
生成一个新的区块
"""
block = {
'index': index,
'timestamp': timestamp,
'data': data,
'proof': proof,
'previous_hash': previous_hash,
}
return block
```
接下来,我们可以在类的初始化方法中创建Genesis块:
```python
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
"""
创建创世区块
"""
return generate_block(0, time.time(), 'Hello World!', 0, '0')
```
在这个方法中,我们调用了生成块的函数,传入了参数0作为索引、当前时间戳、"Hello World!"作为初始数据、0作为证明值、"0"作为前一个块的哈希值,创建了创世块。
这样就完成了块生成函数和Genesis块的创建。
阅读全文
相关推荐
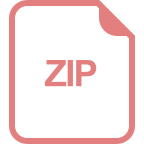
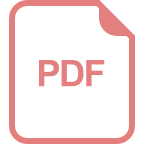
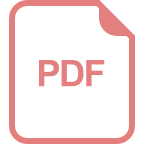
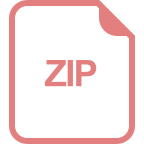
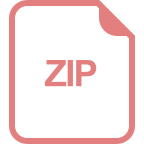
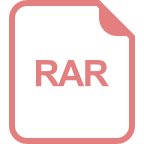
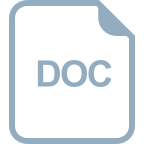
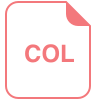
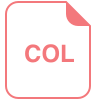
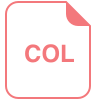




