arduino ide 用esp32-cam做一个蓝牙无线监控的代码
时间: 2024-03-23 19:38:43 浏览: 158
好的,以下是一个基本的 Arduino 代码,用于将 ESP32-CAM 连接到蓝牙并实现无线监控:
```c++
#include "esp_camera.h"
#include <BluetoothSerial.h>
// Define the camera pins
#define CAM_PIN_PWDN 32
#define CAM_PIN_RESET -1
#define CAM_PIN_XCLK 0
#define CAM_PIN_SIOD 26
#define CAM_PIN_SIOC 27
#define CAM_PIN_D7 35
#define CAM_PIN_D6 34
#define CAM_PIN_D5 39
#define CAM_PIN_D4 36
#define CAM_PIN_D3 21
#define CAM_PIN_D2 19
#define CAM_PIN_D1 18
#define CAM_PIN_D0 5
#define CAM_PIN_VSYNC 25
#define CAM_PIN_HREF 23
#define CAM_PIN_PCLK 22
// Create a BluetoothSerial object
BluetoothSerial SerialBT;
void setup() {
Serial.begin(115200);
// Initialize the camera
camera_config_t config;
config.ledc_channel = LEDC_CHANNEL_0;
config.ledc_timer = LEDC_TIMER_0;
config.pin_d0 = CAM_PIN_D0;
config.pin_d1 = CAM_PIN_D1;
config.pin_d2 = CAM_PIN_D2;
config.pin_d3 = CAM_PIN_D3;
config.pin_d4 = CAM_PIN_D4;
config.pin_d5 = CAM_PIN_D5;
config.pin_d6 = CAM_PIN_D6;
config.pin_d7 = CAM_PIN_D7;
config.pin_xclk = CAM_PIN_XCLK;
config.pin_pclk = CAM_PIN_PCLK;
config.pin_vsync = CAM_PIN_VSYNC;
config.pin_href = CAM_PIN_HREF;
config.pin_sscb_sda = CAM_PIN_SIOD;
config.pin_sscb_scl = CAM_PIN_SIOC;
config.pin_pwdn = CAM_PIN_PWDN;
config.pin_reset = CAM_PIN_RESET;
config.xclk_freq_hz = 20000000;
config.pixel_format = PIXFORMAT_JPEG;
if (psramFound()) {
config.frame_size = FRAMESIZE_UXGA;
config.jpeg_quality = 10;
config.fb_count = 2;
} else {
config.frame_size = FRAMESIZE_SVGA;
config.jpeg_quality = 12;
config.fb_count = 1;
}
esp_err_t err = esp_camera_init(&config);
if (err != ESP_OK) {
Serial.printf("Camera init failed with error 0x%x", err);
return;
}
// Initialize the BluetoothSerial object
SerialBT.begin("ESP32-CAM");
}
void loop() {
// Capture a frame from the camera
camera_fb_t *fb = esp_camera_fb_get();
if (!fb) {
Serial.println("Camera capture failed");
return;
}
// Send the frame over Bluetooth
SerialBT.write(fb->buf, fb->len);
// Release the frame buffer
esp_camera_fb_return(fb);
// Wait 50ms before capturing the next frame
delay(50);
}
```
此代码使用 ESP32-CAM 模块连接到蓝牙,并从摄像头捕获图像,并通过蓝牙发送它们。请注意,此代码假定您已经将 ESP32-CAM 配置为连接到 WiFi 并具有 Internet 访问权限。如果您还没有完成此操作,您需要先完成此操作。
阅读全文
相关推荐
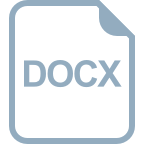
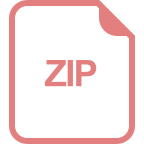
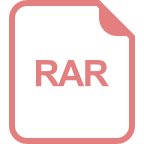















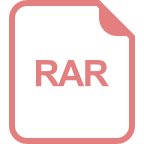