arduino的esp32-cam建立网页视频流教程以及相应的库
时间: 2023-11-16 17:04:51 浏览: 67
要实现Arduino的ESP32-CAM建立网页视频流,可以参考以下步骤:
1. 首先,需要安装ESP32-CAM库,可以在Arduino IDE中搜索并安装该库。
2. 在代码中引入必要的库,如WiFi、AsyncTCP、ESPAsyncWebServer和esp_camera。
3. 设置WiFi连接信息,并连接WiFi。
4. 初始化相机,设置分辨率、帧率等参数。
5. 创建ESPAsyncWebServer实例,并设置路由。
6. 在路由中处理视频流请求,通过调用相机API获取图像数据,将其转换为JPEG格式,并以流的形式发送到客户端。
以下是示例代码:
```
#include <WiFi.h>
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include "esp_camera.h"
// Replace with your network credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Create AsyncWebServer object on port 80
AsyncWebServer server(80);
// Initialize camera
void init_camera() {
camera_config_t config;
config.ledc_channel = LEDC_CHANNEL_0;
config.ledc_timer = LEDC_TIMER_0;
config.pin_d0 = 5;
config.pin_d1 = 18;
config.pin_d2 = 19;
config.pin_d3 = 21;
config.pin_d4 = 36;
config.pin_d5 = 39;
config.pin_d6 = 34;
config.pin_d7 = 35;
config.pin_xclk = 0;
config.pin_pclk = 22;
config.pin_vsync = 25;
config.pin_href = 23;
config.pin_sscb_sda = 26;
config.pin_sscb_scl = 27;
config.pin_pwdn = 32;
config.pin_reset = -1;
config.xclk_freq_hz = 20000000;
config.pixel_format = PIXFORMAT_JPEG;
config.frame_size = FRAMESIZE_VGA;
config.jpeg_quality = 10;
config.fb_count = 2;
// Camera init
esp_err_t err = esp_camera_init(&config);
if (err != ESP_OK) {
Serial.printf("Camera init failed with error 0x%x", err);
return;
}
}
// Serve video stream
void serve_video_stream(AsyncWebServerRequest *request) {
Serial.println("Video stream requested");
if (request->hasParam("frame")) {
// Get JPEG image from camera
camera_fb_t * fb = esp_camera_fb_get();
if (!fb) {
Serial.println("Camera capture failed");
request->send(500, "text/plain", "Camera capture failed");
return;
}
// Send JPEG image as stream
AsyncWebServerResponse *response = request->beginResponse("image/jpeg", fb->len, [fb](uint8_t *buffer, size_t maxLen, size_t index) -> size_t {
if (!index) {
memcpy(buffer, fb->buf, fb->len);
}
return fb->len;
});
response->addHeader("Cache-Control", "no-store");
request->send(response);
// Release the memory
esp_camera_fb_return(fb);
}
else {
request->send(404);
}
}
void setup() {
Serial.begin(115200);
// Connect to Wi-Fi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Initialize camera
init_camera();
// Serve video stream
server.on("/video_stream", HTTP_GET, serve_video_stream);
// Start server
server.begin();
}
void loop() {
}
```
在浏览器中输入ESP32-CAM的IP地址,加上“/video_stream?frame”,即可看到视频流。
注意,由于ESP32-CAM的处理能力有限,视频流可能会有卡顿或延迟,需要根据实际情况调整分辨率、帧率等参数。
相关推荐
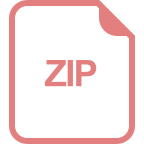
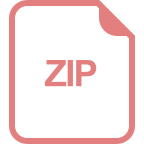














