matlab实现霍夫曼树编码
时间: 2023-10-14 20:06:40 浏览: 108
霍夫曼树编码是一种常见的数据压缩算法,可以利用树形结构对数据进行压缩。下面是使用MATLAB实现霍夫曼树编码的基本步骤:
1. 统计字符出现频率,并根据频率构造霍夫曼树
2. 根据霍夫曼树生成编码表,其中每个字符对应的编码是其在霍夫曼树中的路径
3. 将编码表与需要压缩的数据进行匹配,将数据转换为编码后的二进制字符串
4. 将二进制字符串转换为字节流,即将每8位二进制数转换为一个字节
下面是一个简单的MATLAB实现:
```matlab
function huffman_encoding(input_string, output_file)
% input_string: 待压缩的字符串
% output_file: 压缩后输出的文件
% 统计字符出现频率
freq = hist(double(input_string), 0:255);
% 构造霍夫曼树
nodes = cell(256, 1);
for i = 1:256
nodes{i} = struct('char', i-1, 'freq', freq(i), 'left', [], 'right', []);
end
while length(nodes) > 1
[~, idx] = sort(cellfun(@(x) x.freq, nodes));
left_node = nodes{idx(1)};
right_node = nodes{idx(2)};
new_node = struct('char', [], 'freq', left_node.freq + right_node.freq, 'left', left_node, 'right', right_node);
nodes = [nodes(3:end); {new_node}];
end
% 生成编码表
code_table = cell(256, 1);
for i = 1:256
node = nodes{1};
code = '';
while ~isempty(node.char)
if node.left.char == i-1
code = [code, '0'];
node = node.left;
elseif node.right.char == i-1
code = [code, '1'];
node = node.right;
else
error('Unexpected character');
end
end
code_table{i} = code(end:-1:1);
end
% 将输入字符串转换为编码后的二进制字符串
binary_str = '';
for i = 1:length(input_string)
binary_str = [binary_str, code_table{input_string(i)+1}];
end
% 将二进制字符串转换为字节流
binary_str = [binary_str, repmat('0', [1, mod(-length(binary_str), 8)])];
byte_stream = reshape(binary_str, 8, [])';
byte_stream = bin2dec(byte_stream);
% 写入输出文件
fid = fopen(output_file, 'w');
fwrite(fid, byte_stream, 'uint8');
fclose(fid);
end
```
这个函数接受一个输入字符串和一个输出文件名,将输入字符串压缩并写入输出文件中。可以使用如下方式调用:
```matlab
input_str = 'hello world';
output_file = 'compressed.bin';
huffman_encoding(input_str, output_file);
```
这将会将字符串`'hello world'`压缩并写入文件`'compressed.bin'`中。
阅读全文
相关推荐
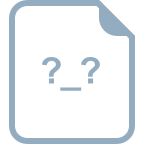
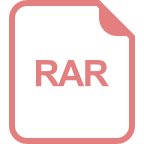
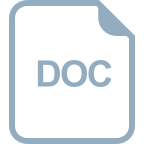
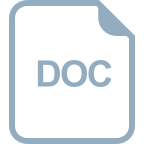
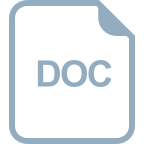
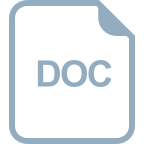
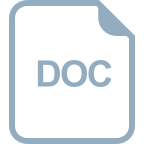
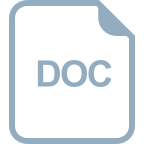
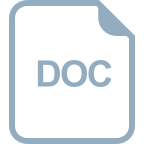
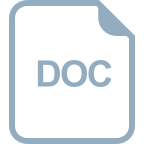
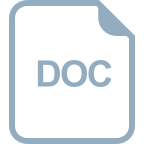
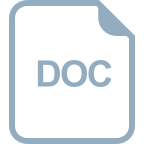
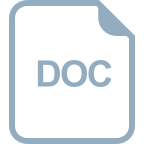

