const eventSource = new EventSource('/oauth/authorize'); // Listen for the 'message' event eventSource.addEventListener('message', event => { // Parse the event data const data = JSON.parse(event.data); // Check if the flag is 1 if (data.flag === 1) { // Redirect to the /oauth/get-user-info page window.location.href = '/oauth/get-user-info'; } }); // Listen for the 'error' event eventSource.addEventListener('error', error => { // Handle the error console.log(error); });
时间: 2024-03-29 19:41:07 浏览: 119
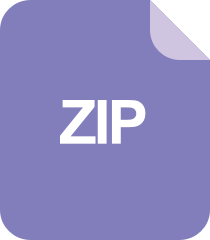
EventSource:提供polyfill以在不可用的浏览器中支持EventSource
这段代码是用来监听一个服务器发送的事件源(EventSource)。这个事件源在服务器端的地址是/oauth/authorize。代码使用了EventSource API并添加了两个监听器。第一个监听器是用来处理服务器发送的消息事件(message),在这个监听器里面,代码将接收到的数据解析成JSON格式,并检查flag是否为1。如果flag为1,代码将会重定向到/oauth/get-user-info页面。第二个监听器是用来处理错误事件(error),当发生错误时,代码会将错误打印出来(通过控制台console.log)。
阅读全文
相关推荐
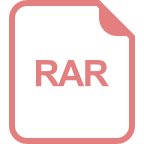
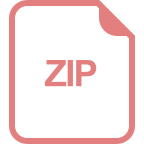
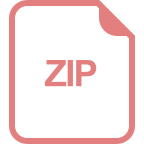
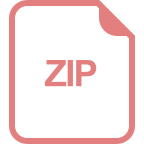
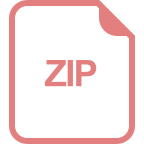
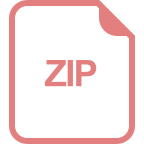
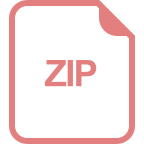
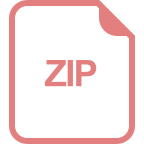
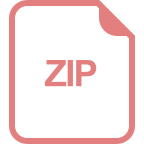
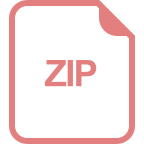
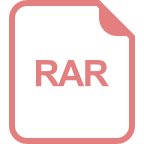






