请根据这段代码import javax.swing.JOptionPane; public class DialogExample { public static void main(String[] args) { StringBuilder contentBuilder = new StringBuilder(); // 在循环中添加新内容 for (int i = 1; i <= 10; i++) { String newContent = "这是第 " + i + " 行内容\n"; contentBuilder.append(newContent); } // 将所有内容输出到对话框中 JOptionPane.showMessageDialog(null, contentBuilder.toString()); } }进行优化
时间: 2024-02-14 20:13:17 浏览: 17
你可以考虑将循环中添加新内容和将所有内容输出到对话框中的操作分离出来,这样就可以在循环中输出新内容了。具体来说,你可以这样修改代码:
```java
import javax.swing.JOptionPane;
public class DialogExample {
public static void main(String[] args) {
StringBuilder contentBuilder = new StringBuilder();
// 在循环中添加新内容,并输出到对话框
for (int i = 1; i <= 10; i++) {
String newContent = "这是第 " + i + " 行内容\n";
contentBuilder.append(newContent);
JOptionPane.showMessageDialog(null, contentBuilder.toString());
}
}
}
```
这样,每次循环都会输出新的内容到对话框,而不是等到循环结束后再输出所有内容。
相关问题
通过在地址栏输入URL地址,可以访问相应的网页,包括:一个菜单栏(文件、编辑、视图)、两个工具栏、带有滚动条的JEditorPane。菜单栏中,文件的热键是F,包括另存为(热键A,快捷键CTRL+S)和退出(热键1,快捷键CTRL+E)两个菜单项:编辑的热键是E,包括后退 (快捷键CTRL+Z)和前进(快捷键CTRL+D)两个菜单项,视图的热键是V,包括全屏(快捷键CTRL+U)、查看源代码(热键C,快捷键CTRL+C)、刷新 (快捷键CTRL+R)。一个工具栏包括三个按钮:另存为、后退、前进、查看源代码、退出,另外一个工具栏包括一个label(地址),一个文本框JTextField和一个按钮 (转向)另存为(将正在访问的页面进行保存)、前进(访问现有页面的上一个页面)、后退 (访问现有页面的下一个页面)、查看源文件 (查看访问页面的HTML源文件、并提供保存、退出功能) 在地址栏输入URL地址后,可以利用javax.swing.JEditorPane来展示网页内容(可调用setPage(String url)方法),另存为网页可以利用输入/输出流实现 (可将字符串形式的URL地址可以打包成java.net.URL实例,调用其中的openStream())可将网页转换为流,然后通过输入流读取获得源代码,输出流进行保存网页。根据以上内容给我对应的代码,功能尽量完善
好的,以下是一份简单的 Java 代码示例,实现了您提到的功能:
```java
import java.awt.BorderLayout;
import java.awt.Desktop;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
import java.util.Stack;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JEditorPane;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JToolBar;
import javax.swing.KeyStroke;
import javax.swing.SwingConstants;
import javax.swing.SwingUtilities;
public class WebBrowser extends JFrame {
private static final long serialVersionUID = 1L;
private JEditorPane editorPane;
private JLabel urlLabel;
private JButton goButton, backButton, forwardButton, refreshButton, sourceButton;
private JMenuItem saveMenuItem, exitMenuItem, backMenuItem, forwardMenuItem, viewSourceMenuItem, fullScreenMenuItem, refreshMenuItem;
private Stack<String> backStack = new Stack<>();
private Stack<String> forwardStack = new Stack<>();
public WebBrowser() {
super("Web Browser");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setLayout(new BorderLayout());
// 创建菜单栏
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
fileMenu.setMnemonic(KeyEvent.VK_F);
saveMenuItem = new JMenuItem(new SaveAction());
saveMenuItem.setMnemonic(KeyEvent.VK_A);
saveMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_S, ActionEvent.CTRL_MASK));
exitMenuItem = new JMenuItem("Exit", KeyEvent.VK_X);
exitMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_E, ActionEvent.CTRL_MASK));
exitMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
fileMenu.add(saveMenuItem);
fileMenu.addSeparator();
fileMenu.add(exitMenuItem);
JMenu editMenu = new JMenu("Edit");
editMenu.setMnemonic(KeyEvent.VK_E);
backMenuItem = new JMenuItem("Back", KeyEvent.VK_B);
backMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_Z, ActionEvent.CTRL_MASK));
backMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
goBack();
}
});
forwardMenuItem = new JMenuItem("Forward", KeyEvent.VK_F);
forwardMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_D, ActionEvent.CTRL_MASK));
forwardMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
goForward();
}
});
editMenu.add(backMenuItem);
editMenu.add(forwardMenuItem);
JMenu viewMenu = new JMenu("View");
viewMenu.setMnemonic(KeyEvent.VK_V);
fullScreenMenuItem = new JMenuItem("Full Screen", KeyEvent.VK_U);
fullScreenMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_U, ActionEvent.CTRL_MASK));
fullScreenMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
setExtendedState(JFrame.MAXIMIZED_BOTH);
}
});
viewSourceMenuItem = new JMenuItem("View Source", KeyEvent.VK_C);
viewSourceMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_C, ActionEvent.CTRL_MASK));
viewSourceMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
viewSource();
}
});
refreshMenuItem = new JMenuItem("Refresh", KeyEvent.VK_R);
refreshMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_R, ActionEvent.CTRL_MASK));
refreshMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
refresh();
}
});
viewMenu.add(fullScreenMenuItem);
viewMenu.add(viewSourceMenuItem);
viewMenu.add(refreshMenuItem);
menuBar.add(fileMenu);
menuBar.add(editMenu);
menuBar.add(viewMenu);
setJMenuBar(menuBar);
// 创建工具栏
JToolBar toolBar1 = new JToolBar(SwingConstants.HORIZONTAL);
toolBar1.setFloatable(false);
backButton = new JButton(new ImageIcon("back.png"));
backButton.setToolTipText("Back");
backButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
goBack();
}
});
forwardButton = new JButton(new ImageIcon("forward.png"));
forwardButton.setToolTipText("Forward");
forwardButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
goForward();
}
});
refreshButton = new JButton(new ImageIcon("refresh.png"));
refreshButton.setToolTipText("Refresh");
refreshButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
refresh();
}
});
sourceButton = new JButton(new ImageIcon("source.png"));
sourceButton.setToolTipText("View Source");
sourceButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
viewSource();
}
});
toolBar1.add(backButton);
toolBar1.add(forwardButton);
toolBar1.add(refreshButton);
toolBar1.add(sourceButton);
JToolBar toolBar2 = new JToolBar(SwingConstants.HORIZONTAL);
toolBar2.setFloatable(false);
urlLabel = new JLabel("URL: ");
JTextField urlField = new JTextField();
urlField.setPreferredSize(new Dimension(400, 30));
urlField.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String url = urlField.getText();
if (!url.startsWith("http")) {
url = "http://" + url;
}
loadPage(url);
}
});
goButton = new JButton("Go");
goButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String url = urlField.getText();
if (!url.startsWith("http")) {
url = "http://" + url;
}
loadPage(url);
}
});
toolBar2.add(urlLabel);
toolBar2.add(urlField);
toolBar2.add(goButton);
// 创建 JEditorPane
editorPane = new JEditorPane();
editorPane.setEditable(false);
editorPane.setContentType("text/html");
editorPane.setPreferredSize(new Dimension(800, 600));
editorPane.addHyperlinkListener(new HyperlinkListener() {
public void hyperlinkUpdate(HyperlinkEvent e) {
if (e.getEventType() == HyperlinkEvent.EventType.ACTIVATED) {
String url = e.getURL().toString();
loadPage(url);
}
}
});
// 添加组件到窗口
JPanel toolBarPanel = new JPanel(new BorderLayout());
toolBarPanel.add(toolBar1, BorderLayout.NORTH);
toolBarPanel.add(toolBar2, BorderLayout.SOUTH);
add(toolBarPanel, BorderLayout.NORTH);
add(editorPane, BorderLayout.CENTER);
setVisible(true);
}
private void loadPage(String url) {
try {
URL u = new URL(url);
URLConnection conn = u.openConnection();
InputStream in = conn.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
reader.close();
editorPane.setText(sb.toString());
urlLabel.setText("URL: " + url);
backStack.push(url);
forwardStack.clear();
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Failed to load page: " + e.getMessage());
}
}
private void goBack() {
if (!backStack.isEmpty()) {
String url = backStack.pop();
forwardStack.push(url);
if (!backStack.isEmpty()) {
url = backStack.peek();
loadPage(url);
}
}
}
private void goForward() {
if (!forwardStack.isEmpty()) {
String url = forwardStack.pop();
backStack.push(url);
loadPage(url);
}
}
private void refresh() {
if (!backStack.isEmpty()) {
String url = backStack.peek();
loadPage(url);
}
}
private void viewSource() {
if (!backStack.isEmpty()) {
String url = backStack.peek();
try {
URL u = new URL(url);
InputStream in = u.openStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
reader.close();
String content = sb.toString();
String fileName = u.getHost() + u.getPath() + ".html";
FileOutputStream out = new FileOutputStream(fileName);
out.write(content.getBytes());
out.close();
Desktop.getDesktop().open(new File(fileName));
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Failed to view source: " + e.getMessage());
}
}
}
private class SaveAction extends AbstractAction {
private static final long serialVersionUID = 1L;
public SaveAction() {
super("Save As");
putValue(Action.MNEMONIC_KEY, KeyEvent.VK_A);
putValue(Action.ACCELERATOR_KEY, KeyStroke.getKeyStroke(KeyEvent.VK_S, ActionEvent.CTRL_MASK));
}
public void actionPerformed(ActionEvent e) {
if (!backStack.isEmpty()) {
String url = backStack.peek();
try {
URL u = new URL(url);
InputStream in = u.openStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line + "\n");
}
reader.close();
String content = sb.toString();
String fileName = u.getHost() + u.getPath() + ".html";
FileOutputStream out = new FileOutputStream(fileName);
out.write(content.getBytes());
out.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(WebBrowser.this, "Failed to save page: " + ex.getMessage());
}
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new WebBrowser();
}
});
}
}
```
需要注意的是,上述代码中的菜单栏、工具栏和 JEditorPane 的图标可能需要替换为您自己的图片文件,以便正确显示。此外,代码中的网页保存功能实现比较简单,只是将网页内容保存到了同名的 .html 文件中,可能需要根据实际需求进行修改。
校园宿舍管理系统java+swing+jdbc代码
以下是一个简单的校园宿舍管理系统的Java代码,使用了Swing和JDBC库。请注意,这只是一个简单的示例代码,您需要根据自己的需求进行修改和完善。
```java
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
import javax.swing.*;
public class DormitoryManagementSystem extends JFrame implements ActionListener {
JLabel title, nameLabel, genderLabel, roomNoLabel, dateLabel;
JTextField nameField, roomNoField, dateField;
JComboBox genderBox;
JButton addBtn, viewBtn, clearBtn;
Connection conn = null;
PreparedStatement pst = null;
ResultSet rs = null;
public static void main(String[] args) {
new DormitoryManagementSystem();
}
public DormitoryManagementSystem() {
super("校园宿舍管理系统");
Container container = getContentPane();
container.setLayout(new GridLayout(6, 2));
title = new JLabel("宿舍管理系统", JLabel.CENTER);
nameLabel = new JLabel("姓名", JLabel.CENTER);
genderLabel = new JLabel("性别", JLabel.CENTER);
roomNoLabel = new JLabel("房间号", JLabel.CENTER);
dateLabel = new JLabel("入住日期(yyyy-mm-dd)", JLabel.CENTER);
nameField = new JTextField();
genderBox = new JComboBox(new String[] { "男", "女" });
roomNoField = new JTextField();
dateField = new JTextField();
addBtn = new JButton("添加");
viewBtn = new JButton("查看");
clearBtn = new JButton("清空");
addBtn.addActionListener(this);
viewBtn.addActionListener(this);
clearBtn.addActionListener(this);
container.add(title);
container.add(new JLabel());
container.add(nameLabel);
container.add(nameField);
container.add(genderLabel);
container.add(genderBox);
container.add(roomNoLabel);
container.add(roomNoField);
container.add(dateLabel);
container.add(dateField);
container.add(addBtn);
container.add(viewBtn);
container.add(clearBtn);
setSize(400, 250);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/dormitory", "root", "password");
} catch (Exception e) {
System.out.println(e);
}
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addBtn) {
String name = nameField.getText();
String gender = genderBox.getSelectedItem().toString();
String roomNo = roomNoField.getText();
String date = dateField.getText();
try {
pst = conn.prepareStatement("insert into student(name, gender, roomNo, date) values(?,?,?,?)");
pst.setString(1, name);
pst.setString(2, gender);
pst.setString(3, roomNo);
pst.setString(4, date);
pst.executeUpdate();
JOptionPane.showMessageDialog(this, "添加成功");
} catch (SQLException ex) {
System.out.println(ex);
JOptionPane.showMessageDialog(this, "添加失败");
}
} else if (e.getSource() == viewBtn) {
try {
pst = conn.prepareStatement("select * from student");
rs = pst.executeQuery();
StringBuilder sb = new StringBuilder();
while (rs.next()) {
sb.append(rs.getString("name")).append("\t");
sb.append(rs.getString("gender")).append("\t");
sb.append(rs.getString("roomNo")).append("\t");
sb.append(rs.getString("date")).append("\n");
}
JOptionPane.showMessageDialog(this, sb.toString());
} catch (SQLException ex) {
System.out.println(ex);
JOptionPane.showMessageDialog(this, "查询失败");
}
} else if (e.getSource() == clearBtn) {
nameField.setText("");
genderBox.setSelectedIndex(0);
roomNoField.setText("");
dateField.setText("");
}
}
}
```
该代码使用了MySQL数据库,需要首先安装MySQL并创建一个名为dormitory的数据库。请将代码中的“password”替换为您的MySQL密码。
该代码实现了一个简单的宿舍管理系统,包括添加学生信息、查看学生信息和清空输入框等功能。您可以根据自己的需求进行修改和扩展。
相关推荐
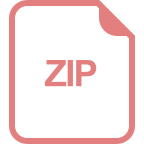












