stringc++push_back()和empty()函数
时间: 2024-09-26 07:05:35 浏览: 51
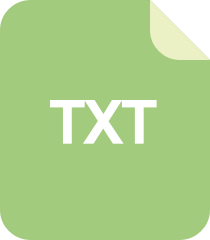
标准c++中string类函数详解
在C++中,`std::string` 是一个动态数组,用于存储字符序列。`push_back()` 和 `empty()` 是它提供的两个常用成员函数:
1. **push_back()** 函数:这个函数用于在字符串的末尾添加单个字符或字符串。它的作用是将指定的元素添加到当前字符串的尾部,并自动调整字符串的大小以容纳新的字符。例如:
```cpp
std::string str;
str.push_back('H');
str.push_back('e');
str.push_back('l');
str.push_back('l');
str.push_back('o');
```
这会使得 `str` 变为 "Hello"。
2. **empty()** 函数:此函数用于检查字符串是否为空,即长度为0。如果字符串没有任何字符,`empty()` 返回 `true`;否则返回 `false`。例如:
```cpp
if (str.empty()) {
std::cout << "The string is empty." << std::endl;
}
```
如果 `str` 仍为初始状态,则 `str.empty()` 会打印出 "The string is empty."
阅读全文
相关推荐
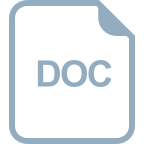
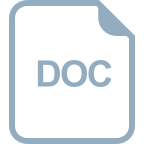


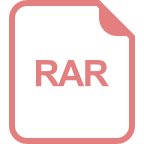
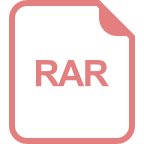
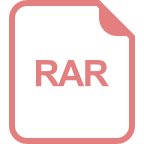
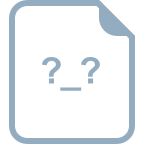
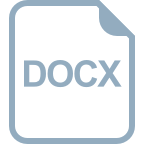
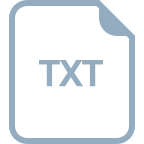
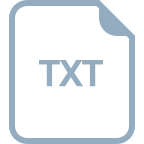
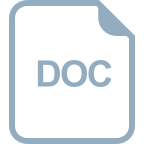





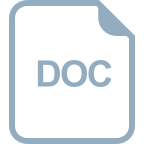