使用c++实现,map<int,map<int,int>>的赋值,和释放
时间: 2023-05-20 18:04:42 浏览: 93
赋值:
```c
#include <map>
int main() {
std::map<int, std::map<int, int>> myMap;
myMap[1][2] = 3;
myMap[4][5] = 6;
// ...
return 0;
}
```
释放:
```c
#include <map>
int main() {
std::map<int, std::map<int, int>> myMap;
// ...
myMap.clear();
return 0;
}
```
注意,`std::map` 会自动管理内存,不需要手动释放。
相关问题
c++ 给std::map<int,std::string>赋值
可以使用 insert 函数或者 [] 运算符来给 std::map<int,std::string> 赋值。具体实现如下:
```c++
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<int, std::string> myMap;
// 使用 insert 函数赋值
myMap.insert(std::make_pair(1, "hello"));
myMap.insert(std::make_pair(2, "world"));
// 使用 [] 运算符赋值
myMap[3] = "C++";
// 遍历 map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
输出结果为:
```
1: hello
2: world
3: C++
```
unordered_map<int, int> map;
unordered_map是C++中的容器,用于存储键和对应的值。在这个例子中,unordered_map<int, int> map表示一个将int类型的键映射到int类型的值的unordered_map。通过map[key]的方式可以访问和操作unordered_map中的键值对。在方式一中,使用值传递遍历方式,将map中的键值对依次赋值给kv,并输出kv的first和second(即键和值)。
阅读全文
相关推荐
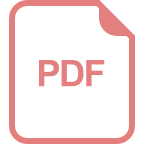
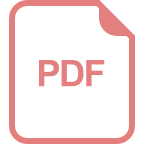





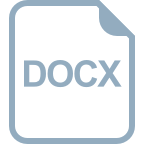







