将实例化出的三个商品对象添加到 goodsList 集合中;
时间: 2024-02-11 07:05:59 浏览: 108
好的,根据你的要求,我理解为你需要在代码中将三个商品对象添加到 `goodsList` 集合中。假设你已经定义了 `Goods` 类,并且实例化出了三个商品对象 `goods1`、`goods2`、`goods3`,可以按照以下步骤将它们添加到 `goodsList` 集合中:
1. 首先要确保 `goodsList` 已经被定义并且实例化了,例如:
```java
List<Goods> goodsList = new ArrayList<>();
```
2. 然后,使用 `add` 方法将三个商品对象添加到 `goodsList` 集合中,例如:
```java
goodsList.add(goods1);
goodsList.add(goods2);
goodsList.add(goods3);
```
这样,就可以将实例化出的三个商品对象添加到 `goodsList` 集合中。
相关问题
我有三个vue组件,分别是goodslist商品主页,goodsdetail01和goodsdetail02商品详情图,我要goodslist在vue.app中显示,实现点击主页goodslist图可以跳转到商品详情页图,不能用路由,用动态组件实现
好的,你可以在 Vue 的 template 部分将三个组件通过动态组件进行切换。可以在 Vue 实例中定义一个变量 `currentComponent`,用于存储当前组件的名称。然后在 `goodslist` 组件中,通过点击事件来修改 `currentComponent` 变量的值,从而实现组件的动态切换。
例如,你可以这样实现:
```html
<template>
<div>
<button @click="currentComponent = 'goodsdetail01'">查看商品详情01</button>
<button @click="currentComponent = 'goodsdetail02'">查看商品详情02</button>
<component :is="currentComponent"></component>
</div>
</template>
<script>
import GoodsDetail01 from './GoodsDetail01.vue'
import GoodsDetail02 from './GoodsDetail02.vue'
export default {
name: 'GoodsList',
components: {
GoodsDetail01,
GoodsDetail02
},
data() {
return {
currentComponent: 'GoodsDetail01'
}
}
}
</script>
```
这样,当你点击 `查看商品详情01` 按钮时,`currentComponent` 变量就会变成 `GoodsDetail01`,从而渲染出 `GoodsDetail01` 组件。同理,当你点击 `查看商品详情02` 按钮时,`currentComponent` 变量就会变成 `GoodsDetail02`,从而渲染出 `GoodsDetail02` 组件。
用java编写。1建个商品类,属性有商品编号、商品名称、商品价格、商品数量 2将商品放入集合中 3执行程序时,有3个功能让用户选择,添加商品、删除商品、显示已有商品。删除商品时需判断商品是否存在(可通过商品编号或者商品名称判断),这三个功能均写成方法。用户选择退出时才能退出。
以下是Java代码实现:
```java
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Scanner;
public class GoodsManagement {
private static List<Goods> goodsList = new ArrayList<>(); // 商品集合
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int choice = 0;
while (choice != 4) {
System.out.println("1.添加商品");
System.out.println("2.删除商品");
System.out.println("3.显示已有商品");
System.out.println("4.退出");
System.out.print("请输入您的选择:");
choice = sc.nextInt();
switch (choice) {
case 1:
addGoods();
break;
case 2:
deleteGoods();
break;
case 3:
showGoods();
break;
case 4:
System.out.println("谢谢使用!");
break;
default:
System.out.println("输入错误,请重新输入!");
}
}
}
// 添加商品
public static void addGoods() {
Scanner sc = new Scanner(System.in);
System.out.print("请输入商品编号:");
int id = sc.nextInt();
System.out.print("请输入商品名称:");
String name = sc.next();
System.out.print("请输入商品价格:");
double price = sc.nextDouble();
System.out.print("请输入商品数量:");
int quantity = sc.nextInt();
Goods goods = new Goods(id, name, price, quantity);
goodsList.add(goods);
System.out.println("商品添加成功!");
}
// 删除商品
public static void deleteGoods() {
Scanner sc = new Scanner(System.in);
System.out.println("请选择删除方式:");
System.out.println("1.按商品编号删除");
System.out.println("2.按商品名称删除");
System.out.print("请输入您的选择:");
int choice = sc.nextInt();
if (choice == 1) {
System.out.print("请输入商品编号:");
int id = sc.nextInt();
boolean flag = false;
Iterator<Goods> it = goodsList.iterator();
while (it.hasNext()) {
Goods goods = it.next();
if (goods.getId() == id) {
it.remove();
flag = true;
System.out.println("商品删除成功!");
break;
}
}
if (!flag) {
System.out.println("商品不存在!");
}
} else if (choice == 2) {
System.out.print("请输入商品名称:");
String name = sc.next();
boolean flag = false;
Iterator<Goods> it = goodsList.iterator();
while (it.hasNext()) {
Goods goods = it.next();
if (goods.getName().equals(name)) {
it.remove();
flag = true;
System.out.println("商品删除成功!");
break;
}
}
if (!flag) {
System.out.println("商品不存在!");
}
} else {
System.out.println("输入错误,请重新选择!");
}
}
// 显示商品
public static void showGoods() {
System.out.println("商品编号\t商品名称\t商品价格\t商品数量");
for (Goods goods : goodsList) {
System.out.println(goods.getId() + "\t\t" + goods.getName() + "\t\t" + goods.getPrice() + "\t\t" + goods.getQuantity());
}
}
}
class Goods {
private int id; // 商品编号
private String name; // 商品名称
private double price; // 商品价格
private int quantity; // 商品数量
public Goods(int id, String name, double price, int quantity) {
this.id = id;
this.name = name;
this.price = price;
this.quantity = quantity;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
```
使用时,直接运行 `GoodsManagement` 类即可。
阅读全文
相关推荐
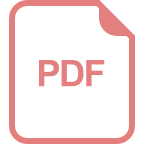
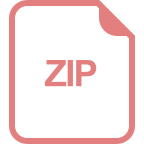
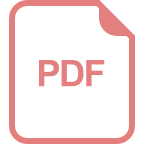










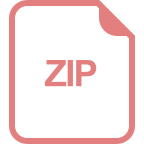