分别利用数组和map来完成如下任务:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数;
时间: 2024-06-08 08:12:17 浏览: 80
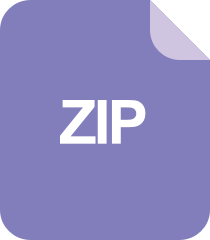
for-of:ES6迭代“ for of”的数组,字符串,数字扩展
利用数组的实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[100];
int cnt[4] = {0}; // 统计英文字母、空格、数字和其它字符的个数
cout << "请输入一行字符:";
cin.getline(str, 100);
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z' || str[i] >= 'A' && str[i] <= 'Z')
cnt[0]++;
else if (str[i] == ' ')
cnt[1]++;
else if (str[i] >= '0' && str[i] <= '9')
cnt[2]++;
else
cnt[3]++;
}
cout << "英文字母个数:" << cnt[0] << endl;
cout << "空格个数:" << cnt[1] << endl;
cout << "数字个数:" << cnt[2] << endl;
cout << "其它字符个数:" << cnt[3] << endl;
return 0;
}
```
利用 map 的实现:
```cpp
#include <iostream>
#include <map>
using namespace std;
int main() {
string str;
map<char, int> cnt;
cout << "请输入一行字符:";
getline(cin, str);
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str[i];
if (ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z')
cnt['A']++;
else if (ch == ' ')
cnt['B']++;
else if (ch >= '0' && ch <= '9')
cnt['C']++;
else
cnt['D']++;
}
cout << "英文字母个数:" << cnt['A'] << endl;
cout << "空格个数:" << cnt['B'] << endl;
cout << "数字个数:" << cnt['C'] << endl;
cout << "其它字符个数:" << cnt['D'] << endl;
return 0;
}
```
阅读全文
相关推荐
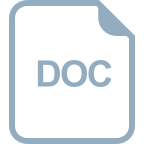
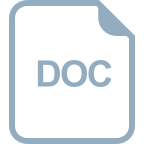















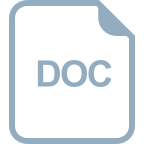