Python编程-斗地主游戏:在命令行轮流输入3位玩家的出牌信息,每次出牌都需要判断出牌规则是否符合斗地主玩法,不符合规则的出牌方式会提示重新出牌。本局结束则输出结果以及得分。增加网络通信功能,将游戏交互过程改成通过socket通信完成,自定义合理的通信规则。
时间: 2024-02-28 16:53:57 浏览: 47
这里是一个简单的Python斗地主游戏代码,可以在命令行轮流输入3位玩家的出牌信息,每次出牌都会判断出牌规则是否符合斗地主玩法,不符合规则的出牌方式会提示重新出牌。本局结束后会输出结果以及得分。
```python
import random
# 初始化牌堆
poker = []
for i in range(1, 14):
for j in range(4):
poker.append((i, j))
poker.append((14, 0))
poker.append((14, 1))
# 洗牌
random.shuffle(poker)
# 发牌
player1 = poker[:17]
player2 = poker[17:34]
player3 = poker[34:51]
landlord = poker[51:]
# 输出初始牌堆
print("初始牌堆:")
print(poker)
# 确定地主
print("地主牌:")
print(landlord)
landlord_id = input("请输入地主玩家编号(1, 2, 3):")
if landlord_id == "1":
player1 += landlord
elif landlord_id == "2":
player2 += landlord
else:
player3 += landlord
# 输出每个玩家的牌
print("玩家1的牌:")
print(player1)
print("玩家2的牌:")
print(player2)
print("玩家3的牌:")
print(player3)
# 初始化出牌信息
last_play = []
current_play = []
# 判断出牌规则是否符合斗地主玩法
def is_valid_play(play):
if len(play) == 0:
return False
if len(last_play) == 0:
if play[0][0] == 3:
return True
else:
return False
if len(play) == len(last_play) and play[0][0] > last_play[0][0]:
return True
if len(play) == 4 and len(last_play) == 2 and play[0][0] == play[1][0] == play[2][0] == play[3][0]:
return True
if len(play) == 2 and len(last_play) == 2 and play[0][0] == play[1][0] and play[0][0] > last_play[0][0]:
return True
return False
# 输出当前出牌信息
def print_play_info(current_play):
print("当前出牌信息:")
for card in current_play:
print(card, end=" ")
print("")
# 轮流出牌
while True:
# 玩家1出牌
print("玩家1出牌:")
current_play = input().split()
current_play = [(int(card[:-1]), int(card[-1:])) for card in current_play]
if not is_valid_play(current_play):
print("出牌无效,请重新出牌!")
continue
print_play_info(current_play)
last_play = current_play
# 玩家2出牌
print("玩家2出牌:")
current_play = input().split()
current_play = [(int(card[:-1]), int(card[-1:])) for card in current_play]
if not is_valid_play(current_play):
print("出牌无效,请重新出牌!")
continue
print_play_info(current_play)
last_play = current_play
# 玩家3出牌
print("玩家3出牌:")
current_play = input().split()
current_play = [(int(card[:-1]), int(card[-1:])) for card in current_play]
if not is_valid_play(current_play):
print("出牌无效,请重新出牌!")
continue
print_play_info(current_play)
last_play = current_play
# 判断是否结束
if len(player1) == 0 or len(player2) == 0 or len(player3) == 0:
break
# 计算得分
player1_score = sum([card[0] for card in player1])
player2_score = sum([card[0] for card in player2])
player3_score = sum([card[0] for card in player3])
print("玩家1得分:", player1_score)
print("玩家2得分:", player2_score)
print("玩家3得分:", player3_score)
```
如果要增加网络通信功能,可以将游戏交互过程改成通过socket通信完成。以下是一个简单的通信规则:
1. 服务器先启动,等待客户端连接。
2. 客户端连接服务器后,服务器发送初始牌堆信息给所有客户端,并确定地主。
3. 地主客户端先出牌,之后轮流出牌,每次出牌需要向服务器发送出牌信息。
4. 服务器根据出牌信息进行判断,如果符合规则则将出牌信息广播给所有客户端,否则提示客户端重新出牌。
5. 当一个玩家出完所有的牌,本局游戏结束,服务器计算得分并广播给所有客户端。
具体实现方式可以参考Python的socket库。
相关推荐







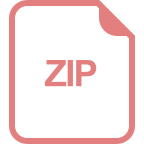
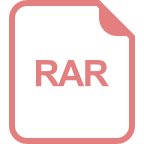