用Python实现斗地主人机对战
时间: 2023-11-05 07:51:28 浏览: 39
斗地主是一种扑克牌游戏,可以使用Python实现人机对战。以下是一个简单的斗地主游戏实现:
```python
import random
# 定义扑克牌花色和点数
suits = ['♠', '♥', '♦', '♣']
ranks = ['3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A', '2', '小王', '大王']
# 创建一副新的扑克牌
deck = [(suit, rank) for suit in suits for rank in ranks]
# 洗牌
random.shuffle(deck)
# 发牌
player1 = deck[:17]
player2 = deck[17:34]
player3 = deck[34:51]
bottom = deck[51:]
# 定义牌型大小顺序
card_order = {'3': 0, '4': 1, '5': 2, '6': 3, '7': 4, '8': 5, '9': 6, '10': 7, 'J': 8, 'Q': 9, 'K': 10, 'A': 11, '2': 12, '小王': 13, '大王': 14}
# 定义出牌函数
def play_card(cards):
if len(cards) == 1:
return True
elif len(cards) == 2 and cards[0][1] == cards[1][1]:
return True
elif len(cards) == 3 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1]:
return True
elif len(cards) == 4 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1]:
return True
elif len(cards) == 5 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1]:
return True
elif len(cards) == 6 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1]:
return True
elif len(cards) == 7 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1]:
return True
elif len(cards) == 8 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1]:
return True
elif len(cards) == 9 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1]:
return True
elif len(cards) == 10 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1] and cards[8][1] == cards[9][1]:
return True
elif len(cards) == 11 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1] and cards[8][1] == cards[9][1] and cards[9][1] == cards[10][1]:
return True
elif len(cards) == 12 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1] and cards[8][1] == cards[9][1] and cards[9][1] == cards[10][1] and cards[10][1] == cards[11][1]:
return True
elif len(cards) == 13 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1] and cards[8][1] == cards[9][1] and cards[9][1] == cards[10][1] and cards[10][1] == cards[11][1] and cards[11][1] == cards[12][1]:
return True
elif len(cards) == 14 and cards[0][1] == cards[1][1] and cards[1][1] == cards[2][1] and cards[2][1] == cards[3][1] and cards[3][1] == cards[4][1] and cards[4][1] == cards[5][1] and cards[5][1] == cards[6][1] and cards[6][1] == cards[7][1] and cards[7][1] == cards[8][1] and cards[8][1] == cards[9][1] and cards[9][1] == cards[10][1] and cards[10][1] == cards[11][1] and cards[11][1] == cards[12][1] and cards[12][1] == cards[13][1]:
return True
else:
return False
# 定义出牌排序函数
def sort_card(cards):
return sorted(cards, key=lambda x: card_order[x[1]])
# 定义人机出牌函数
def ai_play_card(hand_cards, last_cards):
ai_cards = []
if last_cards == []:
# 如果是第一手牌,随机出牌
ai_cards = random.sample(hand_cards, random.randint(1, 3))
else:
# 如果不是第一手牌,按照牌型大小顺序出牌
last_cards_type = len(last_cards)
for i in range(len(hand_cards) - last_cards_type + 1):
if play_card(sort_card(hand_cards[i:i+last_cards_type])):
ai_cards = hand_cards[i:i+last_cards_type]
break
if ai_cards == []:
return []
for card in ai_cards:
hand_cards.remove(card)
return ai_cards
# 游戏开始
print('斗地主游戏开始!')
print('底牌:', bottom)
player1 = sort_card(player1)
player2 = sort_card(player2)
player3 = sort_card(player3)
print('玩家1的手牌:', player1)
print('玩家2的手牌:', player2)
print('玩家3的手牌:', player3)
# 确定地主
landlord = random.randint(1, 3)
if landlord == 1:
player1.extend(bottom)
player1 = sort_card(player1)
print('玩家1成为地主,获得底牌:', bottom)
print('玩家1的手牌:', player1)
elif landlord == 2:
player2.extend(bottom)
player2 = sort_card(player2)
print('玩家2成为地主,获得底牌:', bottom)
print('玩家2的手牌:', player2)
else:
player3.extend(bottom)
player3 = sort_card(player3)
print('玩家3成为地主,获得底牌:', bottom)
print('玩家3的手牌:', player3)
# 出牌
current_player = landlord
last_cards = []
while len(player1) > 0 and len(player2) > 0 and len(player3) > 0:
# 轮流出牌
if current_player == 1:
print('玩家1的手牌:', player1)
played_cards = []
while True:
played_cards = input('请玩家1出牌:').strip().split()
played_cards = [(card[0], card[1:]) for card in played_cards]
if play_card(played_cards) and set(played_cards).issubset(set(player1)):
break
else:
print('出牌不符合规则,请重新出牌!')
for card in played_cards:
player1.remove(card)
last_cards = played_cards
if len(player1) == 0:
print('玩家1获胜!')
break
current_player = 2
elif current_player == 2:
played_cards = ai_play_card(player2, last_cards)
if played_cards == []:
print('玩家2不出!')
else:
print('玩家2出牌:', played_cards)
last_cards = played_cards
if len(player2) == 0:
print('玩家2获胜!')
break
current_player = 3
else:
played_cards = ai_play_card(player3, last_cards)
if played_cards == []:
print('玩家3不出!')
else:
print('玩家3出牌:', played_cards)
last_cards = played_cards
if len(player3) == 0:
print('玩家3获胜!')
break
current_player = 1
```
以上代码仅实现了基本功能,还有很多地方可以优化和改进。
相关推荐
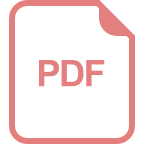
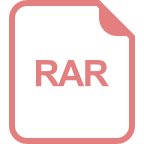
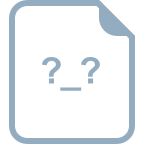
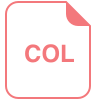
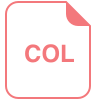
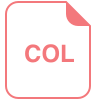
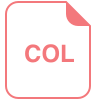
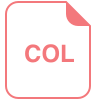










