python围棋人机对战代码
时间: 2024-03-16 08:40:06 浏览: 43
当然,我可以给你介绍一下Python围棋人机对战的代码。在Python中,你可以使用一些库来实现围棋人机对战,比如使用`pygame`库来创建游戏界面,使用`tensorflow`库来构建和训练神经网络模型等。
以下是一个简单的示例代码,展示了如何使用Python实现围棋人机对战:
```python
import pygame
import numpy as np
import tensorflow as tf
# 创建游戏界面
def create_board():
board = np.zeros((19, 19))
return board
# 绘制游戏界面
def draw_board(board):
# 绘制棋盘
for i in range(19):
pygame.draw.line(screen, (0, 0, 0), (30, 30 + i * 30), (570, 30 + i * 30), 1)
pygame.draw.line(screen, (0, 0, 0), (30 + i * 30, 30), (30 + i * 30, 570), 1)
# 绘制棋子
for i in range(19):
for j in range(19):
if board[i][j] == 1:
pygame.draw.circle(screen, (0, 0, 0), (30 + j * 30, 30 + i * 30), 13)
elif board[i][j] == -1:
pygame.draw.circle(screen, (255, 255, 255), (30 + j * 30, 30 + i * 30), 13)
# 人机对战
def human_vs_ai():
board = create_board()
pygame.init()
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("围棋人机对战")
screen.fill((255, 206, 158))
draw_board(board)
pygame.display.flip()
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
x, y = event.pos
i = (y - 30) // 30
j = (x - 30) // 30
if i >= 0 and i < 19 and j >= 0 and j < 19 and board[i][j] == 0:
board[i][j] = 1
draw_board(board)
pygame.display.flip()
# AI下棋
ai_move = ai_play(board)
board[ai_move[0]][ai_move[1]] = -1
draw_board(board)
pygame.display.flip()
clock.tick(60)
pygame.quit()
# AI下棋
def ai_play(board):
# 使用训练好的神经网络模型进行预测
model = tf.keras.models.load_model('model.h5')
# 进行预测...
return move
if __name__ == '__main__':
human_vs_ai()
```
这只是一个简单的示例代码,你可以根据自己的需求进行修改和扩展。同时,你还需要准备一个训练好的神经网络模型来进行AI的下棋预测。
相关推荐
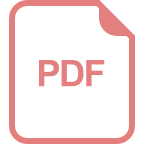
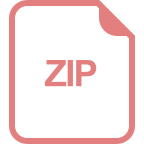














