爱因斯坦棋人机对战代码
时间: 2023-07-09 18:49:30 浏览: 167
以下是一个简单的Python代码示例,可以实现人机对战的爱因斯坦棋游戏:
```python
import random
# 初始化棋盘
board = [
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-'],
['-', '-', '-', '-', '-', '-', '-', '-', '-', '-']
]
# 随机生成初始棋子位置
pieces = [1, 1, 2, 2, 2, 3, 3, 4, 4, 5]
random.shuffle(pieces)
for row in range(10):
for col in range(10):
if (row, col) in [(0, 3), (0, 6), (3, 0), (3, 9), (6, 0), (6, 9), (9, 3), (9, 6)]:
board[row][col] = '*'
elif pieces:
board[row][col] = str(pieces.pop(0))
# 打印初始棋盘
for row in range(10):
for col in range(10):
print(board[row][col], end=' ')
print()
# 人机对战
while True:
# 人类玩家走棋
human_move = input('Your move (e.g. B6-D6): ')
from_row, from_col, to_row, to_col = map(int, human_move.replace('-', ''))
board[to_row][to_col] = board[from_row][from_col]
board[from_row][from_col] = '-'
# 打印新棋盘
for row in range(10):
for col in range(10):
print(board[row][col], end=' ')
print()
# 判断是否获胜
if board[0][4] == '5':
print('You win!')
break
# 电脑玩家走棋
while True:
from_row, from_col = random.randint(0, 9), random.randint(0, 9)
if board[from_row][from_col] != '-':
break
while True:
to_row, to_col = random.randint(0, 9), random.randint(0, 9)
if (to_row, to_col) in [(0, 3), (0, 6), (3, 0), (3, 9), (6, 0), (6, 9), (9, 3), (9, 6)]:
continue
if board[to_row][to_col] == '-':
break
board[to_row][to_col] = board[from_row][from_col]
board[from_row][from_col] = '-'
# 打印新棋盘
for row in range(10):
for col in range(10):
print(board[row][col], end=' ')
print()
# 判断是否获胜
if board[9][4] == '5':
print('Computer wins!')
break
```
这个代码示例可以让人类玩家和电脑玩家轮流走棋,直到有一方获胜。但是请注意,这只是一个简单的示例,它可能不是最优的实现方式。如果您需要更完整、更实用的代码,请考虑使用现成的爱因斯坦棋游戏库或人工智能程序。
阅读全文
相关推荐
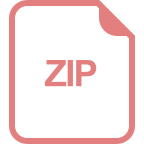
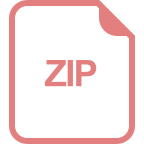
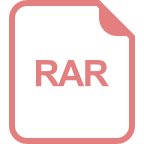
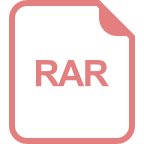
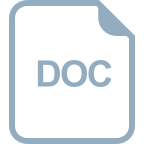
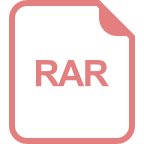
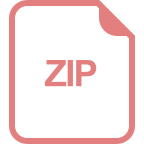
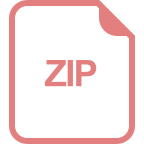
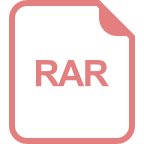
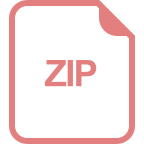
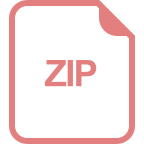
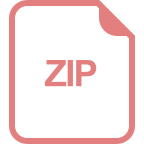
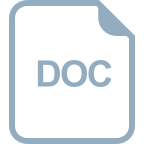
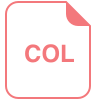
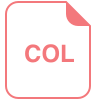