用Python实现五子棋小游戏人机对战
时间: 2023-10-11 20:07:13 浏览: 51
要实现五子棋小游戏人机对战,可以使用Python编写一个简单的图形界面,然后使用算法进行人机对战。
以下是一个基本的实现过程:
1. 导入必要的库
``` python
import tkinter as tk
from tkinter import messagebox
import random
import numpy as np
```
2. 定义棋盘类
``` python
class Chessboard:
def __init__(self, size, spacing):
self.size = size # 棋盘大小
self.spacing = spacing # 线间距
self.chess_size = int(spacing * 0.8) # 棋子大小
self.board = np.zeros((size, size), dtype=np.int) # 记录棋盘状态
self.player = 1 # 玩家标记
```
3. 定义图形界面类
``` python
class GUI:
def __init__(self, size=15, spacing=30):
self.cb = Chessboard(size, spacing)
self.root = tk.Tk()
self.canvas = tk.Canvas(self.root, bg="#F5DEB3", width=spacing*(size+1), height=spacing*(size+1))
self.canvas.pack()
self.draw_board()
self.canvas.bind("<Button-1>", self.click)
self.root.mainloop()
```
4. 绘制棋盘
``` python
def draw_board(self):
for i in range(self.cb.size):
x1, y1, x2, y2 = self.cb.spacing, self.cb.spacing*(i+1), self.cb.spacing*(self.cb.size+1), self.cb.spacing*(i+1)
self.canvas.create_line(x1, y1, x2, y2)
x1, y1, x2, y2 = self.cb.spacing*(i+1), self.cb.spacing, self.cb.spacing*(i+1), self.cb.spacing*(self.cb.size+1)
self.canvas.create_line(x1, y1, x2, y2)
```
5. 定义落子函数
``` python
def drop(self, x, y):
if self.cb.board[x][y] != 0:
return False
self.cb.board[x][y] = self.cb.player
color = "black" if self.cb.player == 1 else "white"
self.canvas.create_oval(self.cb.spacing*(x+1)-self.cb.chess_size, self.cb.spacing*(y+1)-self.cb.chess_size,
self.cb.spacing*(x+1)+self.cb.chess_size, self.cb.spacing*(y+1)+self.cb.chess_size,
fill=color)
return True
```
6. 定义AI落子函数
``` python
def ai_drop(self):
empty_points = np.argwhere(self.cb.board == 0)
if len(empty_points) == 0:
return False
x, y = self.get_best_point(empty_points)
self.drop(x, y)
return True
```
7. 定义胜负判断函数
``` python
def is_win(self, x, y):
player = self.cb.board[x][y]
# 横向判断
count = 1
left, right = y, y
while left > 0 and self.cb.board[x][left-1] == player:
count += 1
left -= 1
while right < self.cb.size-1 and self.cb.board[x][right+1] == player:
count += 1
right += 1
if count >= 5:
return True
# 纵向判断
count = 1
up, down = x, x
while up > 0 and self.cb.board[up-1][y] == player:
count += 1
up -= 1
while down < self.cb.size-1 and self.cb.board[down+1][y] == player:
count += 1
down += 1
if count >= 5:
return True
# 左上到右下判断
count = 1
x1, y1, x2, y2 = x, y, x, y
while x1 > 0 and y1 > 0 and self.cb.board[x1-1][y1-1] == player:
count += 1
x1 -= 1
y1 -= 1
while x2 < self.cb.size-1 and y2 < self.cb.size-1 and self.cb.board[x2+1][y2+1] == player:
count += 1
x2 += 1
y2 += 1
if count >= 5:
return True
# 左下到右上判断
count = 1
x1, y1, x2, y2 = x, y, x, y
while x1 > 0 and y1 < self.cb.size-1 and self.cb.board[x1-1][y1+1] == player:
count += 1
x1 -= 1
y1 += 1
while x2 < self.cb.size-1 and y2 > 0 and self.cb.board[x2+1][y2-1] == player:
count += 1
x2 += 1
y2 -= 1
if count >= 5:
return True
return False
```
8. 定义获胜提示函数
``` python
def show_win(self):
winner = "黑方" if self.cb.player == 1 else "白方"
messagebox.showinfo("游戏结束", winner + "获胜!")
self.root.quit()
```
9. 定义鼠标点击事件函数
``` python
def click(self, event):
x, y = int(round((event.x - self.cb.spacing) / self.cb.spacing)), int(round((event.y - self.cb.spacing) / self.cb.spacing))
if x < 0 or x >= self.cb.size or y < 0 or y >= self.cb.size:
return
if not self.drop(x, y):
return
if self.is_win(x, y):
self.show_win()
else:
self.cb.player = 2
self.ai_drop()
if self.is_win(x, y):
self.show_win()
else:
self.cb.player = 1
```
10. 运行程序
``` python
if __name__ == '__main__':
gui = GUI()
```
以上是一个简单的五子棋小游戏人机对战的实现过程。可以根据实际需要进行修改和优化。
相关推荐
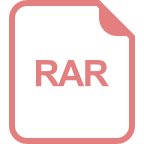














