有一学生类(Student),含有姓名(name)、年龄(age)属性,该类实现Comparable接口,要求重写compareTo方法,实现按照年龄的大小来确定两个学生的大小关系。 在链表中添加3个学生对象,通过Collections类的sort方法对链表中的学生按照年龄升序排序。输入第4个学生对象,并查找他(她)的年龄是否与链表中某个学生的年龄相同。
时间: 2024-03-21 19:44:38 浏览: 12
好的,我知道你的问题了。以下是对应的Java代码:
```
import java.util.Collections;
import java.util.LinkedList;
class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public int compareTo(Student other) {
return Integer.compare(this.age, other.age);
}
}
public class Main {
public static void main(String[] args) {
LinkedList<Student> students = new LinkedList<>();
students.add(new Student("Alice", 20));
students.add(new Student("Bob", 18));
students.add(new Student("Charlie", 22));
Collections.sort(students);
Student newStudent = new Student("David", 20);
boolean found = false;
for (Student s : students) {
if (s.getAge() == newStudent.getAge()) {
found = true;
break;
}
}
if (found) {
System.out.println("Found a student with the same age as David.");
} else {
System.out.println("No student with the same age as David was found.");
}
}
}
```
这段代码首先定义了一个 `Student` 类,实现了 `Comparable` 接口,并重写了 `compareTo` 方法。然后在 `main` 函数中创建了一个链表,并向其中添加了3个学生对象。接着调用 `Collections.sort` 方法,对链表中的学生按照年龄升序排序。
接下来创建了一个新的学生对象 `newStudent`,并在链表中查找是否有年龄和他相同的学生对象。如果找到了,输出提示信息;否则输出另一条提示信息。
希望这段代码能够帮助到你!
相关推荐
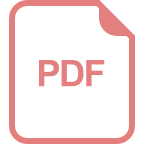
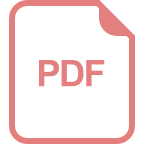














