用C语言写一个算法int Delete_Elem(SqList &L,ElemType e)从L中删除与e相等的数据元素,并返回删除后的L的长度。
时间: 2024-09-16 11:05:14 浏览: 83
在C语言中,你可以使用一个单链表结构(SqList)来编写这个删除元素的算法。首先,我们需要定义链表节点的结构体,以及一个函数来遍历链表、查找元素并进行删除。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
typedef struct Node {
ElemType data; // 数据域
struct Node* next; // 指针域
} Node;
// 定义链表结构体
typedef struct SqList {
Node* head;
int length;
} SqList;
// 初始化链表
void initList(SqList& L) {
L.head = NULL;
L.length = 0;
}
// 插入元素到链表尾部
void insert(SqList& L, const ElemType e) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = e;
newNode->next = L.head;
L.head = newNode;
L.length++;
}
// 删除指定元素
int delete_elem(SqList& L, const ElemType e) {
if (L.head == NULL) return L.length; // 如果链表为空,直接返回长度
Node* curr = L.head;
Node* prev = NULL;
while (curr != NULL && curr->data != e) {
prev = curr;
curr = curr->next;
}
if (curr == NULL) return L.length; // 元素未找到,返回当前长度
if (prev == NULL) { // 如果要删除的是头结点
L.head = curr->next;
} else {
prev->next = curr->next;
}
free(curr); // 释放已删除节点的内存
L.length--; // 减少链表长度
return L.length;
}
阅读全文
相关推荐
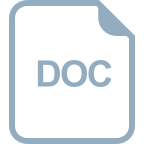
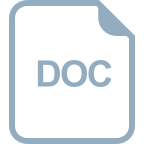
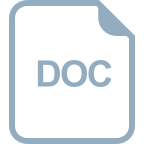















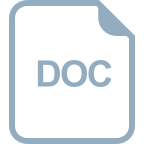