使用标准库,编写stm32f103c8t6通过USART2串口输出数值的程序
时间: 2023-12-18 12:58:01 浏览: 21
以下是一个基本的示例,使用标准库和USART2串口输出一个数值:
```c
#include "stm32f10x.h"
#include <stdio.h>
void USART2_Init(void);
void USART2_SendChar(char ch);
void USART2_SendString(char* str);
void USART2_SendNumber(uint16_t num);
int main(void)
{
// 初始化USART2串口
USART2_Init();
// 待输出的数值
uint16_t num = 1234;
// 将数值转换为字符串并输出到USART2串口
USART2_SendNumber(num);
while(1);
}
void USART2_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能USART2和GPIOA的时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART2的Tx引脚为复用推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART2的参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
// 使能USART2
USART_Cmd(USART2, ENABLE);
}
void USART2_SendChar(char ch)
{
// 等待发送缓冲区为空
while (USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
// 发送字符
USART_SendData(USART2, ch);
}
void USART2_SendString(char* str)
{
// 逐个发送字符
while (*str)
{
USART2_SendChar(*str++);
}
}
void USART2_SendNumber(uint16_t num)
{
char buf[5];
sprintf(buf, "%d", num); // 将数值转换为字符串
USART2_SendString(buf); // 发送字符串
}
```
在这个程序中,我们使用了USART2串口和GPIOA的时钟,并使用`GPIO_InitStructure`和`USART_InitStructure`结构体配置了USART2的参数和Tx引脚的GPIO口。`USART2_Init`函数用于初始化USART2串口。
在`USART2_SendChar`函数中,我们等待发送缓冲区为空,并使用`USART_SendData`函数发送字符。`USART2_SendString`函数逐个发送一个字符串中的字符,而`USART2_SendNumber`函数则将数值转换为字符串并发送。
相关推荐
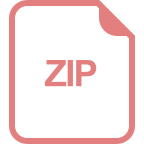
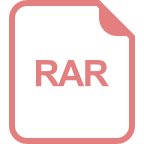







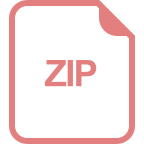
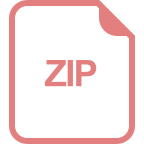
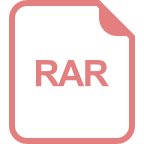
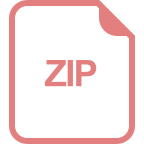
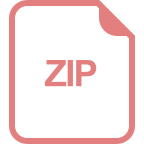