springboot如何实现页面跳转
时间: 2023-07-27 15:23:01 浏览: 96
在 Spring Boot 中,可以使用 Spring MVC 框架来实现页面跳转。要实现页面跳转,可以按照以下步骤:
1. 在控制器(Controller)中添加一个处理请求的方法,例如:
```
@RequestMapping("/index")
public String index() {
return "index";
}
```
2. 在 `resources/templates` 目录下创建一个名为 `index.html` 的 Thymeleaf 模板文件,例如:
```
<!DOCTYPE html>
<html>
<head>
<title>Spring Boot Page</title>
</head>
<body>
<h1>Hello, Spring Boot!</h1>
</body>
</html>
```
3. 当用户访问 `/index` 路径时,控制器会调用 `index()` 方法并返回字符串 `"index"`,Spring MVC 框架会自动解析该字符串并找到对应的模板文件 `index.html`,最终将其渲染并返回给用户。
这样就实现了一个简单的页面跳转。需要注意的是,Spring Boot 默认使用 Thymeleaf 作为模板引擎,因此需要在 `pom.xml` 文件中添加 Thymeleaf 的依赖。
相关问题
springboot实现页面跳转
Spring Boot 可以使用 Thymeleaf 模板引擎来实现页面跳转。首先需要在 pom.xml 文件中添加 Thymeleaf 的依赖,然后在 Controller 中使用 @GetMapping 或 @PostMapping 注解来指定请求的 URL,再返回对应的模板名称即可实现页面跳转。以下是一个简单的示例代码:
```java
@Controller
public class HomeController {
@GetMapping("/")
public String home() {
return "home";
}
}
```
在上面的代码中,@GetMapping("/") 注解指定了请求的 URL 为根路径,然后返回了名为 "home" 的模板名称,Spring Boot 会自动查找名为 "home.html" 的 Thymeleaf 模板文件并渲染出页面。
SpringBoot实现页面跳转重登陆
在 SpringBoot 中实现页面跳转重登录,可以通过使用 Spring Security 框架来实现。下面是实现步骤:
1. 添加 Spring Security 依赖
在 pom.xml 文件中添加 Spring Security 依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 配置 Spring Security
在 SpringBoot 的主配置类中添加 @EnableWebSecurity 注解,并创建一个继承自 WebSecurityConfigurerAdapter 的配置类。通过配置类来指定安全规则和用户权限等信息。
例如,以下代码配置了一个基本的安全规则,即所有请求都需要认证,登录页面为 /login,登录失败后跳转到 /login?error 页面。
```
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/login").failureUrl("/login?error").permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("password").roles("USER");
}
}
```
3. 创建登录页面
在 resources 目录下创建一个名为 login.html 的登录页面,用于用户输入账号和密码。
4. 创建重定向页面
在登录页面中,添加一个重定向链接,如下所示:
```
<a href="/logout?redirect=/myPage">重新登录</a>
```
其中,/logout 是 Spring Security 的默认登出链接,redirect 参数指定了登录后需要跳转的页面。
5. 创建自定义登出处理器
Spring Security 默认的登出处理器不支持重定向到指定页面,需要使用自定义登出处理器来实现。以下代码定义了一个 LogoutHandler 实现类,用于在用户登出时,重定向到指定页面。
```
@Component
public class CustomLogoutHandler implements LogoutSuccessHandler {
@Override
public void onLogoutSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException, ServletException {
String redirectUrl = request.getParameter("redirect");
if (StringUtils.isBlank(redirectUrl)) {
redirectUrl = "/";
}
response.sendRedirect(redirectUrl);
}
}
```
6. 配置自定义登出处理器
在 SpringBoot 的主配置类中,通过 @Bean 注解将自定义登出处理器加入 Spring 容器,并将其设置为 Spring Security 的默认登出处理器。
```
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomLogoutHandler customLogoutHandler;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.logout().logoutSuccessHandler(customLogoutHandler);
// 其他配置
}
// 其他配置
@Bean
public LogoutSuccessHandler logoutSuccessHandler() {
return customLogoutHandler;
}
}
```
7. 测试
完成上述步骤后,重新启动应用程序,访问 /myPage 页面。如果未登录,则会自动跳转到 /login 页面,输入正确的账号和密码后,会重定向到 /myPage 页面。当用户点击重新登录链接时,会跳转到 /login 页面,并在登录成功后重定向到 /myPage 页面。
阅读全文
相关推荐
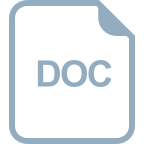
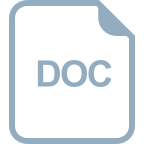
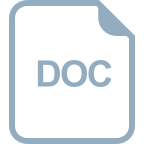
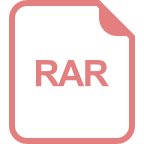












