js httpurl 中 name= 传参占位符
时间: 2024-03-28 22:40:45 浏览: 194
在 HTTP URL 中,传递参数的另一种方式是通过查询字符串(Query String)。查询字符串是 URL 中的一部分,以问号 ? 开头,后面跟着多个键值对,每个键值对之间使用 & 符号分隔。例如:
```
https://example.com/users?name=Alice&age=25
```
这个 URL 中,查询字符串中有两个键值对,分别是 name=Alice 和 age=25。在 JavaScript 中,可以使用 URLSearchParams 对象来解析查询字符串中的参数。例如:
```
const url = "https://example.com/users?name=Alice&age=25";
const params = new URLSearchParams(url.split("?")[1]);
const name = params.get("name");
const age = params.get("age");
console.log(name); // 输出 "Alice"
console.log(age); // 输出 "25"
```
这段代码中,先使用 split() 方法将 URL 分成两部分,即路径部分和查询字符串部分,然后使用 URLSearchParams 对象来解析查询字符串中的参数。get() 方法可以根据参数名获取对应的值。
相关问题
js httpurl 中传参占位符
在 HTTP URL 中,传参占位符通常是用于 RESTful API 的。在 RESTful API 中,URL 中的一部分可以作为参数来传递数据,这些参数通常使用冒号 : 来定义。例如:
```
https://example.com/users/:userId
```
这个 URL 中,:userId 就是一个传参占位符,它可以匹配任意字符串,例如:
```
https://example.com/users/123
https://example.com/users/456
https://example.com/users/abc
```
在 JavaScript 中,可以使用正则表达式来匹配这种传参占位符。例如:
```
const url = "https://example.com/users/123";
const pattern = /\/users\/:([^\/]+)/;
const match = url.match(pattern);
const userId = match[1];
console.log(userId); // 输出 "123"
```
这段代码中,正则表达式 /\/users\/:([^\/]+)/ 可以匹配任意以 /users/ 开头,后面跟着一个传参占位符的 URL。match() 方法可以返回一个数组,其中第一个元素是整个匹配的字符串,后面的元素是每个括号内匹配的字符串。因此,match[1] 就是传参占位符中的实际参数。
js httpurl 中键值对要求 是传参占位符
在 HTTP URL 中,查询字符串的键值对中,键名和键值都是可以任意指定的,不一定要使用传参占位符。如果你想在 URL 中使用传参占位符,可以将占位符作为键名或键值,例如:
```
https://example.com/users?userId=123
https://example.com/users?name=:Alice
```
这些 URL 中,查询字符串中的键名和键值都使用了传参占位符,它们的实际值会在请求时动态替换。在 JavaScript 中,解析这种带有传参占位符的查询字符串和普通的查询字符串是一样的,使用 URLSearchParams 对象即可。例如:
```
const url = "https://example.com/users?userId=123";
const params = new URLSearchParams(url.split("?")[1]);
const userId = params.get("userId");
console.log(userId); // 输出 "123"
const url2 = "https://example.com/users?name=:Alice";
const params2 = new URLSearchParams(url2.split("?")[1]);
const name = params2.get("name");
console.log(name); // 输出 ":Alice"
```
这段代码中,先使用 split() 方法将 URL 分成两部分,然后使用 URLSearchParams 对象来解析查询字符串中的参数。get() 方法可以根据参数名获取对应的值。需要注意的是,这种情况下,获取到的值可能包含传参占位符,需要在后续的处理中将其替换为实际的值。
阅读全文
相关推荐
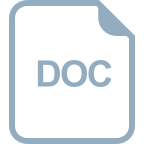
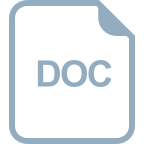
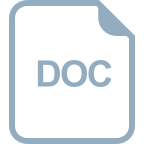
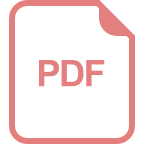




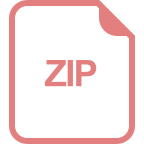
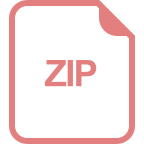
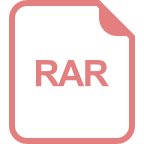
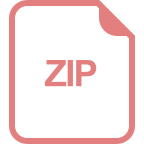
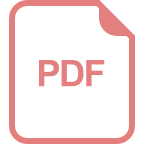
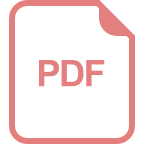

