#include <iostream> #include <fstream> #include <vector> #include <unordered_map> using namespace std; void compress(const string& inputFilePath, const string& outputFilePath) { // 读取输入文件 ifstream inputFile(inputFilePath, ios::binary); // 构建字典 unordered_map<string, int> dictionary; int dictionarySize = 256; for (int i = 0; i < 256; i++) { dictionary[string(1, i)] = i; } vector<int> compressedData; string currentString; char inputChar; while (inputFile.get(inputChar)) { // 获取当前字符串 string newString = currentString + inputChar; // 当前字符串在字典中存在时继续添加字符 if (dictionary.find(newString) != dictionary.end()) { currentString = newString; } // 当前字符串不在字典中时 else { // 将当前字符串添加到压缩数据中 compressedData.push_back(dictionary[currentString]); // 将新的字符串添加到字典中 dictionary[newString] = dictionarySize++; currentString = string(1, inputChar); } } // 处理最后一组字符串 if (!currentString.empty()) { compressedData.push_back(dictionary[currentString]); } // 输出到文件 ofstream outputFile(outputFilePath, ios::binary); for (const int& code : compressedData) { outputFile.write((char*)&code, sizeof(int)); } } int main(int argc, char* argv[]) { if (argc < 3) { cout << "Usage: LZWCompressor <input_file> <output_file>" << endl; } else { string inputFilePath = argv[1]; string outputFilePath = argv[2]; compress(inputFilePath, outputFilePath); } return 0; }如何使用这段代码压缩文件
时间: 2024-02-14 20:13:22 浏览: 138
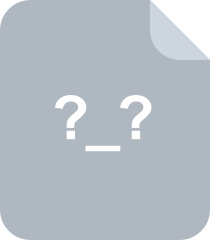
#include <iostream>
你可以按照以下步骤使用这段代码压缩文件:
1. 将代码保存为LZWCompressor.cpp文件。
2. 在命令行中使用以下命令编译代码:
```g++ LZWCompressor.cpp -o LZWCompressor```
3. 在命令行中使用以下命令运行程序并压缩文件:
```./LZWCompressor input_file output_file```
其中,input_file为待压缩的文件路径,output_file为压缩后的文件路径。
4. 程序运行结束后,将生成一个压缩后的文件,文件名为output_file。
阅读全文