5个进程,有进程块,用随机分配和银行家算法 实现分配流程C++代码
时间: 2024-02-27 17:54:50 浏览: 54
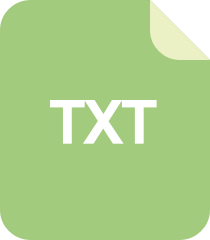
银行家算法 C++实现代码

以下是使用随机分配和银行家算法实现分配流程的C++代码示例:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_PROCESS = 5;
const int MAX_RESOURCE = 3;
int available[MAX_RESOURCE];
int allocation[MAX_PROCESS][MAX_RESOURCE];
int need[MAX_PROCESS][MAX_RESOURCE];
bool finish[MAX_PROCESS];
// 初始化资源数量和分配情况
void init() {
srand(time(NULL));
for (int i = 0; i < MAX_RESOURCE; i++) {
available[i] = rand() % 5 + 1;
for (int j = 0; j < MAX_PROCESS; j++) {
allocation[j][i] = 0;
need[j][i] = rand() % (available[i] + 1);
available[i] -= need[j][i];
}
}
for (int i = 0; i < MAX_PROCESS; i++) {
finish[i] = false;
}
}
// 随机分配算法
void randomAllocate(int pid) {
for (int i = 0; i < MAX_RESOURCE; i++) {
int request = rand() % (need[pid][i] + 1);
available[i] -= request;
allocation[pid][i] += request;
need[pid][i] -= request;
}
}
// 银行家算法
bool bankerAlgorithm(int pid) {
int work[MAX_RESOURCE];
for (int i = 0; i < MAX_RESOURCE; i++) {
work[i] = available[i];
}
bool finishCopy[MAX_PROCESS];
for (int i = 0; i < MAX_PROCESS; i++) {
finishCopy[i] = finish[i];
}
// 尝试分配资源
for (int i = 0; i < MAX_RESOURCE; i++) {
if (need[pid][i] > work[i]) {
return false;
}
work[i] -= need[pid][i];
allocation[pid][i] += need[pid][i];
need[pid][i] = 0;
finishCopy[pid] = true;
}
// 检查是否存在安全序列
bool found;
do {
found = false;
for (int i = 0; i < MAX_PROCESS; i++) {
if (!finishCopy[i]) {
bool canFinish = true;
for (int j = 0; j < MAX_RESOURCE; j++) {
if (need[i][j] > work[j]) {
canFinish = false;
break;
}
}
if (canFinish) {
found = true;
for (int j = 0; j < MAX_RESOURCE; j++) {
work[j] += allocation[i][j];
}
finishCopy[i] = true;
}
}
}
} while (found);
// 检查是否所有进程都能完成
for (int i = 0; i < MAX_PROCESS; i++) {
if (!finishCopy[i]) {
return false;
}
}
return true;
}
int main() {
init();
// 随机分配算法
cout << "Random allocation algorithm:" << endl;
for (int i = 0; i < MAX_PROCESS; i++) {
randomAllocate(i);
}
cout << "Available resources:" << endl;
for (int i = 0; i < MAX_RESOURCE; i++) {
cout << available[i] << " ";
}
cout << endl << endl;
// 银行家算法
cout << "Banker's algorithm:" << endl;
for (int i = 0; i < MAX_PROCESS; i++) {
if (bankerAlgorithm(i)) {
cout << "Process " << i << " finished." << endl;
for (int j = 0; j < MAX_RESOURCE; j++) {
available[j] += allocation[i][j];
}
finish[i] = true;
}
}
cout << "Available resources:" << endl;
for (int i = 0; i < MAX_RESOURCE; i++) {
cout << available[i] << " ";
}
cout << endl;
return 0;
}
```
注意:这只是一个简单的示例,实际使用时需要根据具体情况进行修改和优化。
阅读全文
相关推荐
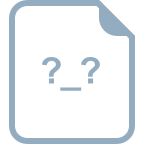

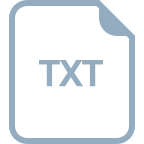
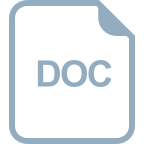

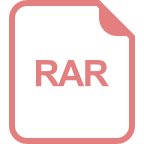
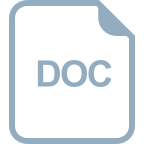
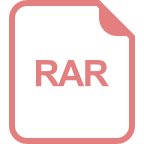
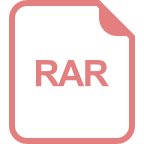
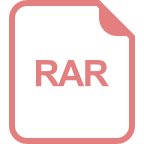
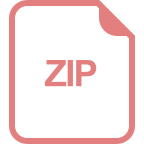
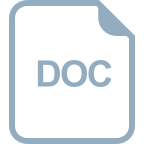
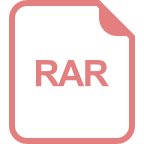
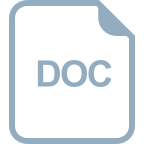
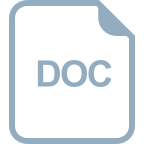

