1.设计一个二次函数,从该函数中采样20个左右的训练数据(可以用随机函数加一些噪声) 2.用matplotlib绘制函数 3.创建一个训练模型,利用上述20个训练样本,用随机梯度下降法训练模型 4.创建一些测试数据,测试训练好的模型。 5.程序用PyTorch实现。
时间: 2024-11-10 16:14:21 浏览: 28
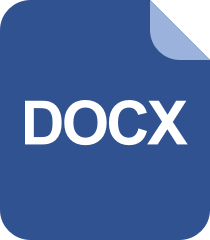
python支持向量机实验任选一个一次函数作为输入,对函数进行采样,并在采样点中加入噪声线性核函数对采样点进行回归
首先,让我们一步步地实现这个程序:
1. **设计二次函数并添加噪声**:
我们可以使用`numpy`库中的`random`模块生成随机数,然后构造一个二次函数 `y = ax^2 + bx + c` 并添加一些随机噪声。
```python
import numpy as np
def generate_data(num_samples, a=1, b=-2, c=0, noise_std=0.1):
x = np.linspace(-10, 10, num_samples) # 生成等间距的x值
y_true = a * x**2 + b * x + c # 函数值
y_noisy = y_true + noise_std * np.random.randn(num_samples) # 添加噪声
return x, y_noisy
```
2. **使用matplotlib绘制函数**:
使用matplotlib画出函数图像:
```python
import matplotlib.pyplot as plt
def plot_function(x, y_noisy):
plt.scatter(x, y_noisy, label='Training Data')
plt.plot(x, a*x**2 + b*x + c, 'r', label='True Function')
plt.legend()
plt.xlabel('x')
plt.ylabel('y')
plt.title('Quadratic Function with Noise')
plt.show()
# 利用之前生成的数据绘制
x, y_noisy = generate_data(20)
plot_function(x, y_noisy)
```
3. **创建训练模型 - 随机梯度下降**:
这里我们假设我们有一个简单的线性模型,实际上二次函数应该用更复杂的模型如多项式回归或神经网络来处理。然而,为了简单起见,我们可以用一个线性模型和随机梯度下降进行模拟。
```python
class LinearModel:
def __init__(self, w1=0, w2=0):
self.w1 = w1
self.w2 = w2
def predict(self, X):
return self.w1 * X + self.w2
def gradient_descent(self, X, y, learning_rate=0.01, num_iterations=1000):
for _ in range(num_iterations):
dw1 = -np.mean(2 * (self.predict(X) - y)) / len(y)
dw2 = -np.mean(2 * (self.predict(X) - y) * X) / len(y)
self.w1 -= learning_rate * dw1
self.w2 -= learning_rate * dw2
# 创建模型实例并训练
model = LinearModel()
model.gradient_descent(x, y_noisy)
```
4. **创建测试数据并测试模型**:
```python
test_x = np.linspace(-10, 10, 100)
test_y_pred = model.predict(test_x)
plt.figure()
plt.scatter(x, y_noisy, label='Training Data')
plt.plot(test_x, test_y_pred, 'b', label='Predicted Function')
plt.legend()
plt.xlabel('x')
plt.ylabel('y')
plt.title('Testing the Model on Test Data')
plt.show()
```
5. **用PyTorch实现**:
如果你想用PyTorch来实现这个过程,你需要定义一个神经网络层,但因为我们的例子比较简单,直接用线性模型可能更好。以下是一个简化的版本:
```python
import torch
from torch import nn
class LinearRegression(nn.Module):
def __init__(self):
super().__init__()
self.linear = nn.Linear(1, 1) # 假设输入是一维的
def forward(self, x):
return self.linear(x.view(-1))
# 使用PyTorch数据加载器、损失函数和优化器
train_dataset = torch.tensor(np.c_[x, y_noisy], dtype=torch.float)
test_dataset = torch.tensor(np.c_[test_x, np.zeros_like(test_x)], dtype=torch.float)
model = LinearRegression()
criterion = nn.MSELoss() # Mean Squared Error Loss for regression
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
for _ in range(num_iterations):
optimizer.zero_grad()
predictions = model(train_dataset[:, :1]) # 取出特征部分
loss = criterion(predictions, train_dataset[:, 1])
loss.backward()
optimizer.step()
# 测试模型
with torch.no_grad():
test_predictions = model(test_dataset[:, :1])
```
阅读全文
相关推荐
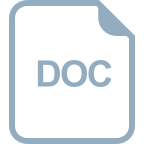
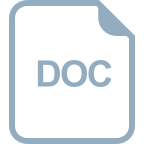

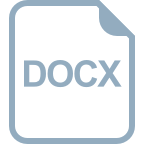
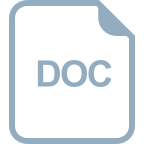
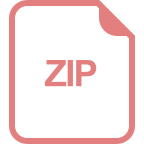
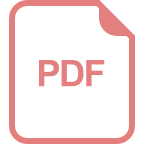
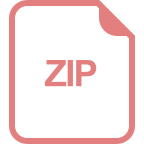
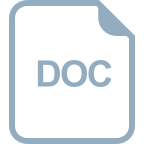
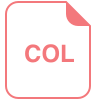
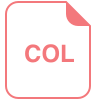
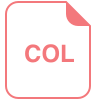
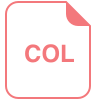
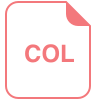
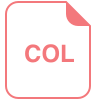
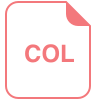
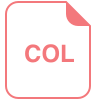

