用Java编写一个圆,圆柱体的类,圆柱体继承圆,求圆面积、圆周长,圆柱体的体积
时间: 2024-05-02 08:23:31 浏览: 122
和表面积。
好的,这是一个具体的编程问题,我可以帮你解答。你可以定义一个圆的类,其中包含圆的半径属性和圆的面积、周长的方法,然后再定义一个圆柱体的类,它继承了圆,拥有圆柱的高度属性,再通过继承圆的面积和周长方法,计算出圆柱体的体积和表面积。以下是可能的实现代码:
```java
class Circle {
protected double radius;
Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public double getCircumference() {
return 2 * Math.PI * radius;
}
}
class Cylinder extends Circle {
protected double height;
Cylinder(double radius, double height) {
super(radius);
this.height = height;
}
public double getVolume() {
return getArea() * height;
}
public double getSurfaceArea() {
return 2 * super.getArea() + getCircumference() * height;
}
}
```
在这个例子中,Circle 类有一个成员变量 radius,以及计算面积和周长的方法。Cylinder 类继承了 Circle,并添加了一个成员变量 height,以及计算体积和表面积的方法。getCircumference() 方法使用了 super 关键字来调用继承自 Circle 的方法。
使用这两个类,你可以这样调用它们:
```java
Circle c = new Circle(2.0);
System.out.println("Circle area: " + c.getArea());
System.out.println("Circle circumference: " + c.getCircumference());
Cylinder cy = new Cylinder(2.0, 4.0);
System.out.println("Cylinder volume: " + cy.getVolume());
System.out.println("Cylinder surface area: " + cy.getSurfaceArea());
```
输出结果分别为:
```
Circle area: 12.566370614359172
Circle circumference: 12.566370614359172
Cylinder volume: 50.26548245743669
Cylinder surface area: 62.83185307179586
```
阅读全文
相关推荐
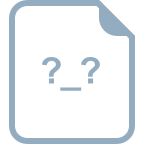















