js如何输出数组中出现次数最多的值
时间: 2024-10-21 15:02:05 浏览: 11
在JavaScript中,你可以使用数组的一些内置方法和额外的数据结构如 Map 或者对象来找出数组中出现次数最多的值。以下是几种常见的解决方案:
**方法一:使用Map和reduce**
```javascript
function maxFrequency(arr) {
let map = new Map();
arr.forEach(item => {
if (map.has(item)) {
map.set(item, map.get(item) + 1);
} else {
map.set(item, 1);
}
});
return Array.from(map.entries()).reduce((a, b) => b[1] > a[1] ? b : a)[0];
}
let array = [1, 2, 3, 2, 2, 4, 5, 2];
console.log(maxFrequency(array)); // 输出:2
```
**方法二:使用对象计数**
```javascript
function mostFrequentValue(arr) {
let count = {};
for (let item of arr) {
if (count[item]) {
count[item]++;
} else {
count[item] = 1;
}
}
return Object.keys(count).reduce((a, b) => count[b] > count[a] ? b : a);
}
let array = [1, 2, 3, 2, 2, 4, 5, 2];
console.log(mostFrequentValue(array)); // 输出:2
```
阅读全文
相关推荐
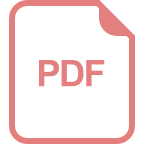
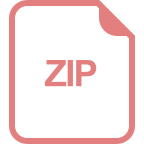
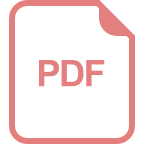


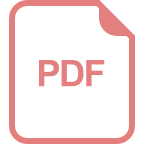
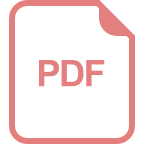
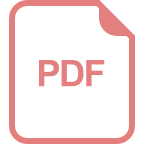
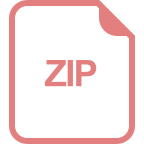
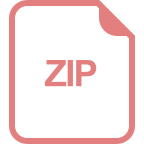




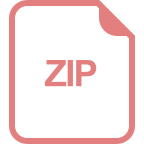

