exercise C程序怎么写
时间: 2024-10-22 11:22:25 浏览: 40
### 解析Exercise C - 最大回文平方数
#### 题目描述
给定一个正整数 \( N \),找到不超过 \( N \) 的最大回文平方数及其平方根 \( M \),满足以下条件:
1. 存在一个正整数 \( M \) 使得 \( M^2 = K \)。
2. \( K \) 的十进制表示是回文数(即从前往后和从后往前读都相同)。
#### 输入
输入一个正整数 \( N \),其中 \( 1 \leq N \leq 10^{12} \)。
#### 输出
输出两个数:最大的回文平方数 \( K \) 和其平方根 \( M \),格式为 `K M`。
#### 示例
- 输入:`490`
- 输出:`484 22`
- 输入:`644406105766`
- 输出:`637832238736 798644`
#### 基础代码
```c
#include <stdio.h>
#include <math.h>
long long reverseNumber(long long x) {
long long revx = 0;
while (x) {
revx = revx * 10 + x % 10;
x /= 10;
}
return revx;
}
int findPalindromicRoot(long long root) {
long long square = root * root;
if (square > N) return -1; // 超过N,返回-1
if (square == reverseNumber(square)) {
return root; // 找到回文平方数
}
return findPalindromicRoot(root - 1); // 继续递归查找
}
int main() {
long long N;
scanf("%lld", &N);
long long root = (long long)sqrt(N); // 从sqrt(N)开始向下查找
long long resultRoot = findPalindromicRoot(root);
if (resultRoot != -1) {
printf("%lld %lld\n", resultRoot * resultRoot, resultRoot);
} else {
printf("No palindromic square found.\n");
}
return 0;
}
```
#### 代码解析
1. **reverseNumber 函数**:用于反转一个数字,判断是否为回文数。
2. **findPalindromicRoot 函数**:递归函数,从给定的 `root` 开始向下查找,直到找到一个满足条件的回文平方数或超过范围。
3. **main 函数**:
- 读取输入的 \( N \)。
- 计算 \( \sqrt{N} \) 作为初始的 `root`。
- 调用 `findPalindromicRoot` 查找最大的回文平方数及其平方根。
- 输出结果。
这个方法通过递归从 \( \sqrt{N} \) 向下查找,确保找到的最大回文平方数不超过 \( N \)。
阅读全文
相关推荐





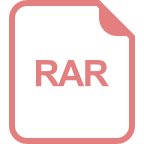









