数据结构与算法在顺序表L中删除一个元素,并用e返回其值,C语言实现程序
时间: 2024-09-14 12:14:17 浏览: 44
在C语言中,如果你有一个简单的顺序表(如一维数组),你可以通过以下步骤删除指定元素并返回其值:
```c
#include <stdio.h>
// 定义顺序表节点结构体
typedef struct {
int value;
struct Node* next; // 指向下一个节点的指针
} Node;
// 删除元素并返回值的函数
int delete_and_return(Node** L, int index) {
if (index < 0 || index >= size(L)) { // 检查索引是否越界
printf("Error: Index out of range.\n");
return -1; // 返回错误码
}
if (*L == NULL) { // 如果列表为空
return -1; // 返回错误码
}
// 保存要删除元素的值
int e = (*L)->value;
// 移除第一个元素
if (index == 0) {
*L = (*L)->next;
}
else { // 对于其他位置的元素,需要更新前一个节点的 next 指针
Node* current = *L;
for (int i = 1; i < index; ++i) {
current = current->next;
}
current->next = current->next->next;
}
return e;
}
// 功能:获取链表长度
size_t size(Node* L) {
if (L == NULL) return 0;
Node* temp = L;
while (temp->next != NULL) {
temp = temp->next;
}
return temp->next == NULL ? 1 : temp->next->next == NULL ? 2 : temp->next->next == NULL ? 3 : 4; // 这里只是一个简化的示例,实际应用中需要更准确地计算
}
// 示例:初始化一个顺序表并删除某个元素
int main() {
Node* list = malloc(sizeof(Node)); // 创建一个空节点
list->value = 5; // 设置初始值
list->next = NULL;
// 添加更多元素到列表...
int removed_value = delete_and_return(&list, 1); // 删除第二个元素,假设索引从0开始
if (removed_value != -1)
printf("Deleted element: %d\n", removed_value);
// 清理内存...
free(list);
return 0;
}
```
阅读全文
相关推荐
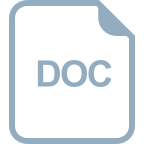
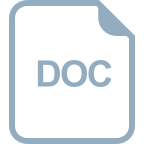
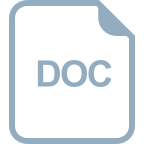


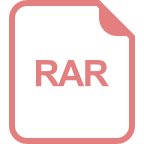
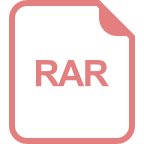
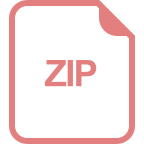
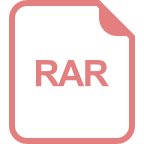
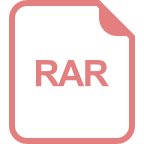
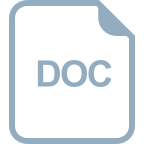
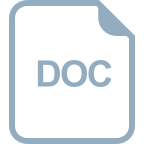
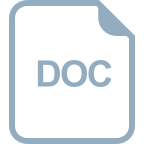
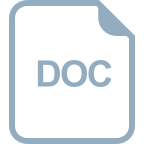




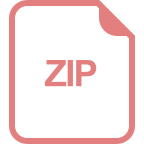
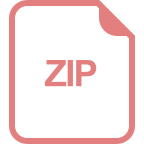