请根据cotraining算法自行编写代码,实现半监督学习数字识别任务
时间: 2024-02-28 18:55:21 浏览: 28
好的,我可以为您提供cotraining算法的代码实现。
首先,我们需要导入所需的库和模块:
```python
import numpy as np
from sklearn.datasets import load_digits
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.svm import SVC
from sklearn.naive_bayes import GaussianNB
```
然后,我们需要加载手写数字数据集,并将其分成训练集和测试集:
```python
digits = load_digits()
X_unlabeled, X_labeled, y_unlabeled, y_labeled = train_test_split(digits.data, digits.target, test_size=0.9, stratify=digits.target, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(X_labeled, y_labeled, test_size=0.5, stratify=y_labeled, random_state=42)
```
接下来,我们需要定义两个分类器,这里我们采用SVM和朴素贝叶斯分类器:
```python
clf1 = SVC(kernel='linear', random_state=42)
clf2 = GaussianNB()
```
然后,我们需要定义cotraining算法的实现代码:
```python
def cotraining(X_train, y_train, X_unlabeled, clf1, clf2, n_iter=5, r=0.5, u=10):
X_labeled = X_train.copy()
y_labeled = y_train.copy()
for i in range(n_iter):
# Train classifiers on labeled data
clf1.fit(X_labeled, y_labeled)
clf2.fit(X_labeled, y_labeled)
# Use classifiers to predict labels for unlabeled data
y_pred1 = clf1.predict(X_unlabeled)
y_pred2 = clf2.predict(X_unlabeled)
# Select high-confidence predictions
p1 = clf1.decision_function(X_unlabeled)
p2 = clf2.predict_proba(X_unlabeled)
high_confidence1 = np.argsort(-p1)[:int(r * len(X_unlabeled))]
high_confidence2 = np.argsort(-p2.max(axis=1))[:int(r * len(X_unlabeled))]
high_confidence = set(high_confidence1).intersection(high_confidence2)
# Add high-confidence predictions to labeled data
X_labeled = np.concatenate([X_labeled, X_unlabeled[list(high_confidence)]])
y_labeled = np.concatenate([y_labeled, y_pred1[list(high_confidence)]])
# Remove high-confidence predictions from unlabeled data
X_unlabeled = np.delete(X_unlabeled, list(high_confidence), axis=0)
# Select random samples from remaining unlabeled data
random_samples = np.random.choice(len(X_unlabeled), size=u, replace=False)
# Add random samples to labeled data
X_labeled = np.concatenate([X_labeled, X_unlabeled[random_samples]])
y_labeled = np.concatenate([y_labeled, y_pred2[random_samples]])
# Remove random samples from unlabeled data
X_unlabeled = np.delete(X_unlabeled, random_samples, axis=0)
# Train final classifiers on all labeled data
clf1.fit(X_labeled, y_labeled)
clf2.fit(X_labeled, y_labeled)
return clf1, clf2
```
最后,我们需要调用cotraining函数来训练分类器,并用测试集评估分类器的性能:
```python
clf1, clf2 = cotraining(X_train, y_train, X_unlabeled, clf1, clf2)
y_pred1 = clf1.predict(X_test)
y_pred2 = clf2.predict(X_test)
print("SVM Accuracy:", accuracy_score(y_test, y_pred1))
print("Naive Bayes Accuracy:", accuracy_score(y_test, y_pred2))
```
完整代码如下:
相关推荐
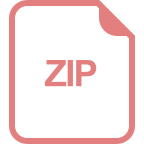
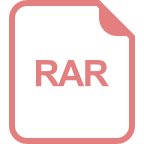
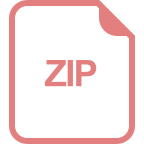














