用java写一段代码,从map判断某个key是否存在,如果不存在,则调用某个方法获取返回值,然后将key和返回值放入map中; 需要考虑并发问题,防止同一个key重复调用这个方法
时间: 2024-12-17 07:46:35 浏览: 1
在Java中,我们可以使用ConcurrentHashMap来处理并发问题,因为ConcurrentHashMap提供线程安全的操作。下面是一个简单的示例,展示了如何检查键是否存在并避免重复调用获取方法:
```java
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicReference;
public class ConcurrentMapExample {
private ConcurrentHashMap<String, AtomicReference<Object>> map = new ConcurrentHashMap<>();
private Object getVal(String key) {
// 这里假设getVal方法是耗时或有状态操作
if (!map.containsKey(key)) {
// 使用AtomicReference存储计算结果,保证可见性和唯一性
map.putIfAbsent(key, new AtomicReference<>(calculateValue(key)));
}
return map.get(key).get();
}
private Object calculateValue(String key) {
// 实现具体的计算逻辑,这里仅做演示
try {
Thread.sleep(1000); // 模拟耗时操作
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Calculated Value for " + key;
}
public void main(String[] args) {
String key1 = "Key1";
String key2 = "Key2";
// 线程A
Runnable threadA = () -> {
if (getVal(key1) == null) {
System.out.println("Thread A calculated and added to map: " + getVal(key1));
}
};
// 线程B
Runnable threadB = () -> {
if (getVal(key2) == null) {
System.out.println("Thread B calculated and added to map: " + getVal(key2));
}
};
new Thread(threadA).start();
new Thread(threadB).start(); // 同时启动两个线程
// 等待所有任务完成
threadA.join();
threadB.join();
}
}
```
在这个例子中,`ConcurrentHashMap`的`putIfAbsent`方法会原子性地检查键是否存在,并在不存在时添加新条目。`AtomicReference`用于存储计算结果,确保在多线程环境下的可见性。
阅读全文
相关推荐
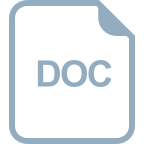
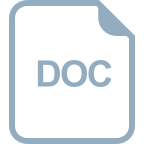
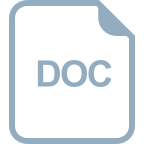















