wdf驱动获取pcie配置空间代码
时间: 2023-10-30 10:05:11 浏览: 32
下面是一个简单的示例代码,用于获取PCIe设备的配置空间:
```c
#include <linux/pci.h>
static int my_probe(struct pci_dev *pdev, const struct pci_device_id *id)
{
u8 val8;
u16 val16;
u32 val32;
int err;
/* 获取Vendor ID 和 Device ID */
u16 vendor_id = pci_read_config_word(pdev, PCI_VENDOR_ID);
u16 device_id = pci_read_config_word(pdev, PCI_DEVICE_ID);
/* 获取Revision ID 和 Class Code */
u8 revision_id = pci_read_config_byte(pdev, PCI_REVISION_ID);
u32 class_code = pci_read_config_dword(pdev, PCI_CLASS_REVISION);
/* 读取配置空间中的一个字节 */
err = pci_read_config_byte(pdev, 0x40, &val8);
if (err) {
dev_err(&pdev->dev, "Could not read byte from config space\n");
return err;
}
/* 读取配置空间中的一个双字节 */
err = pci_read_config_word(pdev, 0x44, &val16);
if (err) {
dev_err(&pdev->dev, "Could not read word from config space\n");
return err;
}
/* 读取配置空间中的一个四字节 */
err = pci_read_config_dword(pdev, 0x48, &val32);
if (err) {
dev_err(&pdev->dev, "Could not read dword from config space\n");
return err;
}
/* 打印读取到的值 */
dev_info(&pdev->dev, "Vendor ID: 0x%04x, Device ID: 0x%04x\n", vendor_id, device_id);
dev_info(&pdev->dev, "Revision ID: 0x%02x, Class Code: 0x%08x\n", revision_id, class_code);
dev_info(&pdev->dev, "Byte at offset 0x40: 0x%02x\n", val8);
dev_info(&pdev->dev, "Word at offset 0x44: 0x%04x\n", val16);
dev_info(&pdev->dev, "Dword at offset 0x48: 0x%08x\n", val32);
return 0;
}
static struct pci_device_id my_pci_tbl[] = {
{ PCI_DEVICE(0x8086, 0x100e) },
{ 0, }
};
MODULE_DEVICE_TABLE(pci, my_pci_tbl);
static struct pci_driver my_pci_driver = {
.name = "my_pci_driver",
.id_table = my_pci_tbl,
.probe = my_probe,
};
module_pci_driver(my_pci_driver);
```
这个代码中,`my_probe()` 函数是PCIe设备的probe函数,用于初始化设备并获取配置空间信息。在这个函数中,我们首先使用 `pci_read_config_xxx()` 函数读取PCIe设备的Vendor ID、Device ID、Revision ID和Class Code等信息,然后分别读取配置空间中的一个字节、一个双字节和一个四字节,并将读取到的值打印出来。
在 `main()` 函数中,我们定义了一个 `my_pci_driver` 结构体,并将其传递给 `module_pci_driver()` 函数以注册驱动程序。`my_pci_tbl` 数组用于指定PCIe设备的Vendor ID和Device ID。
注意,在使用 `pci_read_config_xxx()` 函数时,第一个参数是 `struct pci_dev *pdev`,即指向PCIe设备结构体的指针,第二个参数是配置空间的偏移量(以字节为单位),第三个参数是一个指向存储读取到的值的变量的指针。如果读取成功,这个函数将返回0,否则返回一个负数错误码。
相关推荐
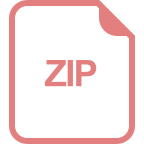
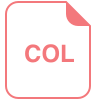
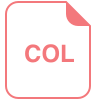
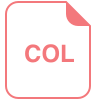
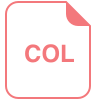
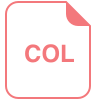









