python中文代码雨
时间: 2023-09-23 10:05:47 浏览: 131
### 回答1:
我可以回答这个问题。Python中文代码雨是一种特殊的代码效果,可以在Python代码中添加中文字符,使代码更加生动有趣。这种效果可以通过在代码中添加注释来实现,例如在代码中添加 # -*- coding: utf-8 -*- 来指定编码格式,然后在注释中添加中文字符即可。
### 回答2:
Python中的“代码雨”是指一种经典的动态效果,表现为屏幕上不断下落的字符组成的代码流。这种效果通常用于展示代码的美观和动感。
要实现Python中的代码雨效果,可以借助pygame等库来完成。下面是一个简单的代码示例:
```python
import pygame
import random
WIDTH = 800
HEIGHT = 600
FPS = 60
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
clock = pygame.time.Clock()
# 随机生成字符、位置、速度和颜色
def create_code():
code = chr(random.randint(33, 126))
x = random.randint(0, WIDTH)
y = random.randint(-100, -10)
speed = random.randint(1, 5)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
return [code, x, y, speed, color]
code_list = []
# 初始化字符列表
for i in range(100):
code_list.append(create_code())
running = True
while running:
clock.tick(FPS)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
# 更新字符位置
for code_item in code_list:
code_item[2] += code_item[3]
if code_item[2] > HEIGHT:
code_item[2] = random.randint(-100, -10)
pygame.draw.rect(screen, (0, 0, 0), (code_item[1], code_item[2], 10, 10))
pygame.draw.rect(screen, code_item[4], (code_item[1], code_item[2], 10, 10))
pygame.draw.rect(screen, (0, 0, 0), (code_item[1]+2, code_item[2]+2, 6, 6))
pygame.draw.rect(screen, code_item[4], (code_item[1]+2, code_item[2]+2, 6, 6))
pygame.draw.rect(screen, (0, 0, 0), (code_item[1]+4, code_item[2]+4, 2, 2))
pygame.draw.rect(screen, code_item[4], (code_item[1]+4, code_item[2]+4, 2, 2))
pygame.display.flip()
pygame.quit()
```
这段代码通过不断更新字符的位置,实现了代码雨的效果。每个字符随机生成,并具有不同的速度和颜色,使用矩形来表示字符的形状。最后通过pygame的图形库来显示和更新屏幕,从而达到代码雨的效果。
### 回答3:
Python中文码雨是一种具有创意和艺术性的编程现象,它将代码以中文形式呈现,并让代码以动态的方式在屏幕上下降,就像雨水一样。下面是一个简单的示例:
```python
import pygame
import random
# 设置屏幕宽高
width = 800
height = 600
# 初始化pygame
pygame.init()
# 创建屏幕对象
screen = pygame.display.set_mode((width, height))
# 设置背景颜色
bg_color = (0, 0, 0)
# 中文字符集
characters = '雨水python代码'
# 创建字体对象
font = pygame.font.Font('msyh.ttc', 20)
# 创建文字列表
texts = []
for i in range(80):
# 随机选择一个中文字符
character = random.choice(characters)
# 创建字体渲染对象
text = font.render(character, True, (255, 255, 255))
# 将文字和其位置加入列表
texts.append([text, random.randint(0, width-20), random.randint(-height, 0)])
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清屏
screen.fill(bg_color)
# 更新和绘制文字
for text in texts:
# 更新文字的位置
text[2] += random.randint(1, 5)
if text[2] > height:
text[2] = random.randint(-height, 0)
text[1] = random.randint(0, width-20)
# 绘制文字
screen.blit(text[0], (text[1], text[2]))
# 刷新屏幕
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这段代码使用了pygame库来创建窗口和处理事件,并利用随机数来控制中文字符的位置和运动方式。通过不断更新字符的位置,实现了字符的下落效果,从而形成了中文代码雨的效果。
阅读全文
相关推荐
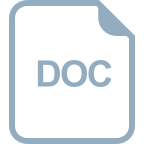
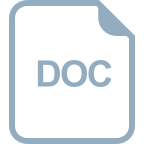
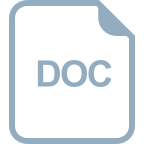
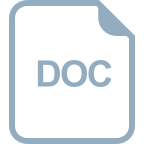
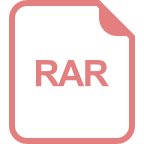
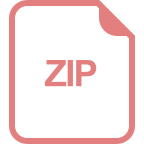
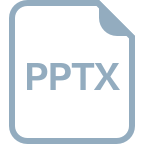
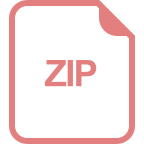
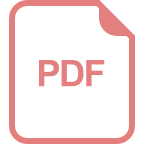
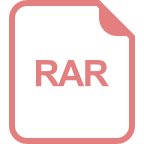
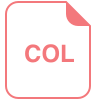




