python orc+坐标 iOS自动化定位
时间: 2024-03-15 10:46:19 浏览: 230
您的问题不太清晰,我猜测您想问的是如何使用 Python 进行 iOS 自动化测试时的 OCR 文字识别和坐标定位。
OCR 文字识别可以使用 Python 的第三方库 pytesseract 来实现,它是一个基于 Google 的开源 OCR 引擎 Tesseract 的 Python 封装。您可以通过以下命令安装:
```
pip install pytesseract
```
在使用 pytesseract 进行 OCR 识别时,您需要先对需要识别的区域进行截图,然后使用 pytesseract.image_to_string() 方法来获取识别结果。例如:
```python
import pytesseract
from PIL import Image
# 截图
im = Image.open('screenshot.png')
region = im.crop((x1, y1, x2, y2))
# OCR 识别
text = pytesseract.image_to_string(region, lang='eng')
print(text)
```
坐标定位可以通过 iOS 自动化测试框架 XCTest 来实现。您可以使用 XCTest 提供的 XCUIElementQuery 来查找您需要的 UI 元素,然后使用 XCUIElement 的坐标信息来进行操作。例如:
```python
import time
import unittest
from appium import webdriver
class iOSAutomationTest(unittest.TestCase):
def setUp(self):
desired_caps = {
"platformName": "iOS",
"platformVersion": "14.5",
"deviceName": "iPhone 12",
"app": "/path/to/your/app",
"automationName": "XCUITest",
"udid": "your-device-udid"
}
self.driver = webdriver.Remote("http://localhost:4723/wd/hub", desired_caps)
def tearDown(self):
self.driver.quit()
def test_example(self):
# 查找 UI 元素
button = self.driver.find_element_by_xpath("//XCUIElementTypeButton[@name='Example']")
# 获取坐标信息
x = button.location['x']
y = button.location['y']
# 点击
self.driver.tap([(x, y)])
# 等待
time.sleep(2)
if __name__ == '__main__':
unittest.main()
```
需要注意的是,iOS 自动化测试需要使用 Appium 作为驱动,并且需要在您的 iOS 设备上安装 Appium 的 WebDriverAgent 服务。同时,您需要在 Appium 中设置正确的 capabilities 和启动参数,才能进行 iOS 自动化测试。
阅读全文
相关推荐
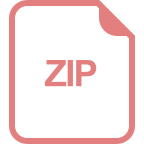
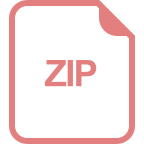
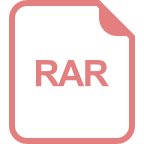
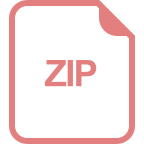
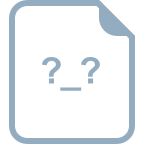
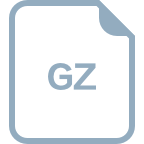







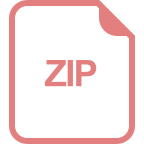