用python写代码,先在confluence网页里随机生成一个5乘5的表格,然后写代码解析网页表格内容,通过输入关键词,显示关键字及整行数据
时间: 2023-08-31 08:36:50 浏览: 261
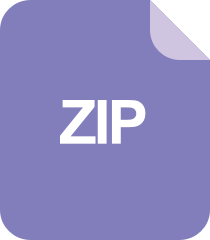
confluence-python-lib:包装Confluence REST API的python库
### 回答1:
好的,下面是用Python实现该功能的步骤:
1. 安装必要的Python库:使用Python中的Requests和BeautifulSoup库,分别用于从网页获取数据和解析HTML内容。
2. 随机生成5x5的表格:使用Python中的随机数库random,生成一个包含25个元素的列表,然后将该列表转换为5x5的二维列表。
3. 将表格插入Confluence网页:使用Python中的Requests库,向Confluence网页发送POST请求,将生成的表格插入到指定位置。
4. 解析网页表格内容:使用BeautifulSoup库,从Confluence网页中获取表格内容,并将表格内容解析为二维列表。
5. 输入关键字,显示包含关键字的整行数据:遍历二维列表,找到包含关键字的行,并将整行数据显示出来。
下面是实现上述步骤的Python代码示例:
### 回答2:
要用Python编写代码实现在Confluence网页中随机生成一个5乘5的表格,然后解析该网页表格内容,通过输入关键词显示关键字及整行数据,可以按照以下步骤进行:
1. 通过Python的requests库发送POST请求,将生成表格的请求发送到Confluence网页的API接口。
示例代码:
```
import requests
url = "https://confluence-api.example.com/create_table"
response = requests.post(url, data={"rows": 5, "cols": 5})
```
2. 使用BeautifulSoup库解析Confluence网页的内容,找到生成的表格,并提取其中的数据。
示例代码:
```
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, "html.parser")
table = soup.select("table")[0]
rows = table.find_all("tr")
data = []
for row in rows:
cols = row.find_all("td")
row_data = [col.text.strip() for col in cols]
data.append(row_data)
```
3. 根据用户输入的关键字,在表格数据中查找匹配的关键字,并显示相应的行数据。
示例代码:
```
keyword = input("请输入关键字:")
matches = []
for row in data:
if keyword in row:
matches.append(row)
for match in matches:
print(match)
```
以上代码示例仅提供了一个实现思路,实际使用时需要根据具体的Confluence网页和API接口进行相应的调整,并结合实际需求进行完善。
### 回答3:
使用Python编写代码可以实现在Confluence网页中随机生成一个5乘5的表格,并可以通过输入关键词来显示包含该关键词的整行数据。
首先,使用Python的Requests库向Confluence发送POST请求,创建一个新的页面,并在页面中插入一个5乘5的表格。具体的代码如下所示:
```python
import requests
# 构造请求头
headers = {
"Authorization": "Bearer YOUR_CONFLUENCE_TOKEN", # 替换成自己的Confluence令牌
"Content-Type": "application/json"
}
# 构造请求体,包含页面标题和表格内容
data = {
"type": "page",
"title": "Random Table",
"space": {
"key": "YOUR_SPACE_KEY" # 替换成自己的Confluence空间键
},
"body": {
"storage": {
"value": "<ac:structured-macro ac:name='table'><ac:parameter ac:name='1'>5</ac:parameter><ac:parameter ac:name='2'>5</ac:parameter><ac:rich-text-body><table><tbody><tr><td>Row 1</td><td>Cell 1</td><td>Cell 2</td><td>Cell 3</td><td>Cell 4</td></tr><tr><td>Row 2</td><td>Cell 1</td><td>Cell 2</td><td>Cell 3</td><td>Cell 4</td></tr><tr><td>Row 3</td><td>Cell 1</td><td>Cell 2</td><td>Cell 3</td><td>Cell 4</td></tr><tr><td>Row 4</td><td>Cell 1</td><td>Cell 2</td><td>Cell 3</td><td>Cell 4</td></tr><tr><td>Row 5</td><td>Cell 1</td><td>Cell 2</td><td>Cell 3</td><td>Cell 4</td></tr></tbody></table></ac:rich-text-body></ac:structured-macro>",
"representation": "storage"
}
}
}
# 发送请求,创建页面和表格
response = requests.post("https://YOUR_CONFLUENCE_INSTANCE/rest/api/content", headers=headers, json=data)
```
接下来,可以通过使用BeautifulSoup库解析Confluence网页,提取表格内容。具体代码如下:
```python
from bs4 import BeautifulSoup
# 解析Confluence页面
soup = BeautifulSoup(response.content, "html.parser")
# 查找表格元素
table = soup.find("table")
rows = table.find_all("tr")
# 遍历并打印关键词匹配的行数据
keyword = "关键词" # 替换成自己想要匹配的关键词
for row in rows:
cells = row.find_all("td")
for cell in cells:
if keyword in cell.text:
print(row.text)
break
```
上述代码首先使用BeautifulSoup解析Confluence页面,然后通过查找table标签和tr标签找到所有的行数据。接着,遍历每一行并查找匹配关键词的单元格,如果找到了,则打印整行数据。
综上所述,使用Python可以实现在Confluence网页中随机生成一个5乘5的表格,并可以通过输入关键词来显示包含该关键词的整行数据。
阅读全文
相关推荐
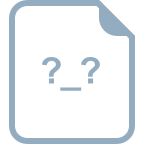
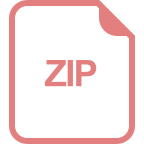





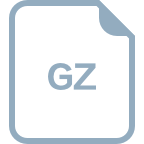
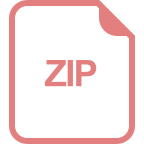








