如何在C++中避免main函数的多重定义?
时间: 2024-09-30 12:12:41 浏览: 75
在C++中避免main函数的多重定义,你可以遵循以下步骤:
1. **将main放在唯一的地方**:确保你的`main()`函数定义只在一个cpp源文件中,通常是程序的入口文件(通常是`main.cpp`)。
2. **使用预处理器指令**:如果你有多个源文件,可以使用`#ifndef ... #define ... #endif`来防止`main()`在多个地方意外定义。例如,在主入口文件顶部:
```cpp
#ifndef _MY_MAIN_
#define _MY_MAIN_()
int main() { ... }
#endif
```
3. **避免循环包含**:确保文件之间的包含关系不会导致main函数被反复声明。如果需要共享代码,考虑使用`include guard`或者公共头文件。
4. **链接时处理**:确保编译生成的目标文件(`.o`或`.obj`)中只包含一个main函数。对于自动生成的项目,比如使用makefile,确认构建规则正确地合并了源文件而不是复制它们。
5. **避免使用框架或库里的main**:除非特别必要,否则不要从库或框架直接使用预定义的main,因为那可能导致意外的定义。
6. **IDE设置**:在集成开发环境(IDE)中,确保项目的构建配置正确,不会在同一编译单元中编译多次main。
通过以上措施,你应该能够避免在C++程序中出现main函数的多重定义问题。
相关问题
写一个c++析构函数含有多重继承与派生
下面是一个含有多重继承与派生的C++程序,其中包含了多个类,每个类都有自己的析构函数实现。
```cpp
#include<iostream>
using namespace std;
class Base1 {
public:
Base1() { cout << "Base1 Constructor" << endl; }
virtual ~Base1() { cout << "Base1 Destructor" << endl; }
};
class Base2 {
public:
Base2() { cout << "Base2 Constructor" << endl; }
virtual ~Base2() { cout << "Base2 Destructor" << endl; }
};
class Derived1 : public Base1 {
public:
Derived1() { cout << "Derived1 Constructor" << endl; }
virtual ~Derived1() { cout << "Derived1 Destructor" << endl; }
};
class Derived2 : public Base2 {
public:
Derived2() { cout << "Derived2 Constructor" << endl; }
virtual ~Derived2() { cout << "Derived2 Destructor" << endl; }
};
class Derived3 : public Derived1, public Derived2 {
public:
Derived3() { cout << "Derived3 Constructor" << endl; }
virtual ~Derived3() { cout << "Derived3 Destructor" << endl; }
};
int main() {
Derived3 d;
return 0;
}
```
上述程序中,我们定义了四个类:`Base1`、`Base2`、`Derived1`和`Derived2`,其中`Derived1`继承自`Base1`,`Derived2`继承自`Base2`。然后我们定义了一个类`Derived3`,它同时从`Derived1`和`Derived2`中派生出来,即实现了多重继承。在`main()`函数中,我们创建了一个`Derived3`的对象`d`,然后程序运行结束时,会自动调用各个类的析构函数进行内存清理。
输出结果如下:
```
Base1 Constructor
Derived1 Constructor
Base2 Constructor
Derived2 Constructor
Derived3 Constructor
Derived3 Destructor
Derived2 Destructor
Base2 Destructor
Derived1 Destructor
Base1 Destructor
```
可以看到,程序运行结束后,各个类的析构函数按照派生关系的顺序依次被调用,完成了内存的清理工作。
用C++生成分别定义教师类Teacher和干部类Cadre,采用多重继承的方式由这两个类派生出新类Teacher_Cadre(教师兼干部类)。要求: (1)在两个基类中都包含姓名、年龄、性别、地址、电话数据成员。 (2)在Teacher类中还包含数据成员职称title,在Cadre类中还包含数据成员职务post,在Teacher_Cadre类中还包含数据成员工资wage。 (3)对两个基类中的姓名、年龄、性别、地址、电话数据成员用相同的名字,在访问这类数据成员时,指定作用域。 (4)在类体中声明成员函数,在类外定义成员函数。 (5)在派生类Teacher_Cadre的成员函数show中调用Teacher类中的display函数,输出姓名、年龄、性别、地址、电话,然后再用cout语句输出职务和工资。
答:好的,以下是代码实现:
```
#include<iostream>
#include<string>
using namespace std;
class Teacher {
public:
string name;
int age;
char gender;
string address;
string phone;
Teacher(string name, int age, char gender, string address, string phone, string title) {
this->name = name;
this->age = age;
this->gender = gender;
this->address = address;
this->phone = phone;
this->title = title;
}
void display() {
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
cout << "地址:" << address << endl;
cout << "电话:" << phone << endl;
}
string title;
};
class Cadre {
public:
string name;
int age;
char gender;
string address;
string phone;
Cadre(string name, int age, char gender, string address, string phone, string post) {
this->name = name;
this->age = age;
this->gender = gender;
this->address = address;
this->phone = phone;
this->post = post;
}
void display() {
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
cout << "地址:" << address << endl;
cout << "电话:" << phone << endl;
}
string post;
};
class Teacher_Cadre : public Teacher, public Cadre {
public:
Teacher_Cadre(string name, int age, char gender, string address, string phone, string title, string post, float wage)
: Teacher(name, age, gender, address, phone, title), Cadre(name, age, gender, address, phone, post) {
this->wage = wage;
}
float wage;
void show() {
Teacher::display();
cout << "职务:" << post << endl;
cout << "工资:" << wage << endl;
}
};
int main() {
Teacher_Cadre tc("小明", 30, '男', "北京市海淀区", "18888888888", "高级讲师", "教务主任", 10000);
tc.show();
return 0;
}
```
解释一下:
首先定义了两个基类 Teacher 和 Cadre,分别包含相同的数据成员和 display 函数。接着定义了要派生的类 Teacher_Cadre,采用多重继承方式同时继承了 Teacher 和 Cadre,增加了工资数据成员和 show 函数。在构造函数中分别调用两个基类的构造函数初始化数据成员,注意需要分别传递两个基类中的数据成员值。在 show 函数中先调用 Teacher 类中的 display 函数输出姓名、年龄、性别、地址和电话,再用 cout 输出职务和工资。
在 main 函数中创建一个 Teacher_Cadre 类型的对象 tc,传递相应的成员数据值,并调用其中的 show 函数,即可输出定义的所有信息。
阅读全文
相关推荐
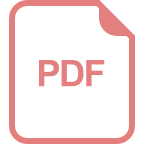
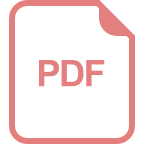
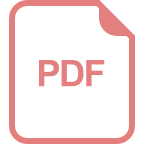
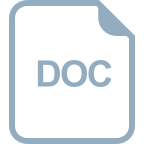
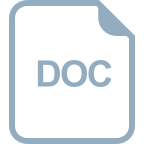
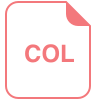
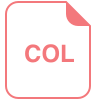
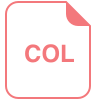
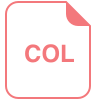
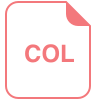






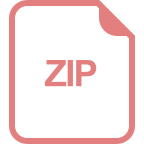