编写C++程序,程序中需要体现类的多重继承、类的多级继承、虚继承、公共继承、保护继承、私有继承、子对象、类的组合、虚函数、纯虚函数等特性,须完整各类的构造函数、析构函数。
时间: 2023-08-10 10:02:56 浏览: 54
好的,我会按照您的要求回答这个问题。以下是一个简单的示例程序:
```c++
#include <iostream>
using namespace std;
// 基类1
class Base1 {
public:
Base1() { cout << "Base1 constructor called." << endl; }
virtual ~Base1() { cout << "Base1 destructor called." << endl; }
};
// 基类2
class Base2 {
public:
Base2() { cout << "Base2 constructor called." << endl; }
virtual ~Base2() { cout << "Base2 destructor called." << endl; }
};
// 派生类1,多重继承自Base1和Base2
class Derived1 : public Base1, public Base2 {
public:
Derived1() { cout << "Derived1 constructor called." << endl; }
virtual ~Derived1() { cout << "Derived1 destructor called." << endl; }
};
// 派生类2,多级继承自Derived1
class Derived2 : public Derived1 {
public:
Derived2() { cout << "Derived2 constructor called." << endl; }
virtual ~Derived2() { cout << "Derived2 destructor called." << endl; }
};
// 派生类3,虚继承自Base1
class Derived3 : virtual public Base1 {
public:
Derived3() { cout << "Derived3 constructor called." << endl; }
virtual ~Derived3() { cout << "Derived3 destructor called." << endl; }
};
// 派生类4,公共继承自Base1
class Derived4 : public Base1 {
public:
Derived4() { cout << "Derived4 constructor called." << endl; }
virtual ~Derived4() { cout << "Derived4 destructor called." << endl; }
};
// 派生类5,保护继承自Base1
class Derived5 : protected Base1 {
public:
Derived5() { cout << "Derived5 constructor called." << endl; }
virtual ~Derived5() { cout << "Derived5 destructor called." << endl; }
};
// 派生类6,私有继承自Base1
class Derived6 : private Base1 {
public:
Derived6() { cout << "Derived6 constructor called." << endl; }
virtual ~Derived6() { cout << "Derived6 destructor called." << endl; }
};
// 子对象
class SubObject {
public:
SubObject() { cout << "SubObject constructor called." << endl; }
virtual ~SubObject() { cout << "SubObject destructor called." << endl; }
};
// 类的组合
class CompositeObject {
public:
CompositeObject() { cout << "CompositeObject constructor called." << endl; }
virtual ~CompositeObject() { cout << "CompositeObject destructor called." << endl; }
private:
SubObject subObj;
};
// 虚函数和纯虚函数
class Base3 {
public:
Base3() { cout << "Base3 constructor called." << endl; }
virtual ~Base3() { cout << "Base3 destructor called." << endl; }
virtual void virtualFunction() { cout << "Base3 virtual function called." << endl; }
virtual void pureVirtualFunction() = 0;
};
class Derived7 : public Base3 {
public:
Derived7() { cout << "Derived7 constructor called." << endl; }
virtual ~Derived7() { cout << "Derived7 destructor called." << endl; }
virtual void virtualFunction() { cout << "Derived7 virtual function called." << endl; }
virtual void pureVirtualFunction() { cout << "Derived7 pure virtual function called." << endl; }
};
int main() {
// 多重继承
Derived1 d1;
// 多级继承
Derived2 d2;
// 虚继承
Derived3 d3;
// 公共继承
Derived4 d4;
// 保护继承
Derived5 d5;
// 私有继承
Derived6 d6;
// 子对象
SubObject sub;
// 类的组合
CompositeObject composite;
// 虚函数和纯虚函数
Base3* ptr = new Derived7;
ptr->virtualFunction();
ptr->pureVirtualFunction();
delete ptr;
return 0;
}
```
在这个示例程序中,我们定义了多个基类和派生类,体现了类的多重继承、类的多级继承、虚继承、公共继承、保护继承、私有继承等特性。我们还定义了子对象和类的组合,以及虚函数和纯虚函数,体现了这些特性的使用方法。同时,在每个类的构造函数和析构函数中,我们输出了一些提示信息,以便观察对象的创建和销毁过程。
相关推荐
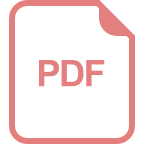
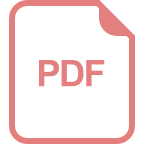
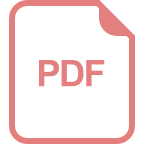



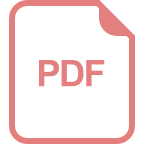
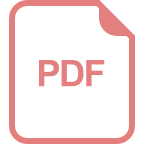
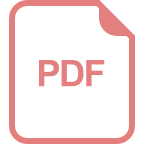
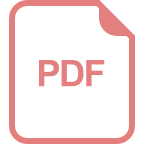
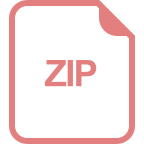