for i in range(polyline.count()):
时间: 2024-01-17 07:39:14 浏览: 111
根据你提供的代码,你正在使用一个名为polyline的对象,并尝试在循环中调用count()方法。如果你遇到了AttributeError: 'Segment' object has no attribute 'count'的错误,意味着polyline对象并没有count()方法。
要解决这个问题,你可以尝试使用其他方法来获取polyline对象的长度或元素数量。例如,你可以使用内置的len()函数来获取对象的长度,像这样:
```python
for i in range(len(polyline)):
# 在这里编写你的代码
```
或者,你可以使用其他与polyline对象相关的方法或属性来获得它的长度。请查阅相关文档或检查polyline对象的定义以获取更多信息。
相关问题
def make_circle(radius=10, res=30, filled=True): points = [] for i in range(res): ang = 2*math.pi*i / res points.append((math.cos(ang)*radius, math.sin(ang)*radius)) if filled: return FilledPolygon(points) else: return PolyLine(points, True)
这段代码定义了一个函数 `make_circle` ,用于创建一个圆形的几何对象。该函数接受三个参数:`radius`(半径,默认为10)、`res`(分辨率,表示圆周上的点的数量,默认为30)、`filled`(是否填充,默认为True)。
函数的实现过程如下:
1. 创建一个空列表 `points` ,用于存储圆周上的点的坐标。
2. 使用循环遍历从0到 `res-1` 的整数,计算每个点在圆周上的角度 `ang` (等分圆周)。
3. 计算每个点的 x 坐标和 y 坐标,分别为 `math.cos(ang)*radius` 和 `math.sin(ang)*radius` ,并将坐标作为元组添加到 `points` 列表中。
4. 如果 `filled` 参数为True,则返回一个填充的多边形对象 `FilledPolygon` ,传入 `points` 列表作为参数。
5. 如果 `filled` 参数为False,则返回一个多段线对象 `PolyLine` ,传入 `points` 列表作为参数,并设置闭合属性为True。
这段代码的作用是创建一个圆形的几何对象,并根据 `filled` 参数决定是否填充该圆形。
def make_circle(radius=10, res=30, filled=True): points = [] for i in range(res): ang = 2*math.pi*i / res points.append((math.cos(ang)*radius, math.sin(ang)*radius)) if filled: return FilledPolygon(points) else: return PolyLine(points, True)
This is a function definition in Python that creates and returns a circle as a polygon or polyline, based on the input parameters.
The `radius` parameter determines the size of the circle, while the `res` parameter controls the number of points used to construct the circle. The more points, the smoother the circle will look.
The `filled` parameter specifies whether the circle should be filled in or just outlined.
The function uses a for loop to generate points on the circle by calculating the x and y coordinates using trigonometry. These points are then stored in a list and returned as either a `FilledPolygon` or `PolyLine` object, depending on the `filled` parameter.
阅读全文
相关推荐
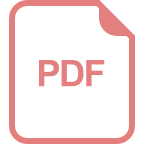
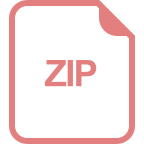
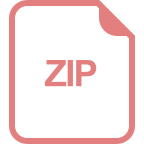
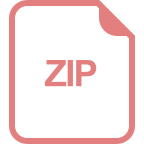
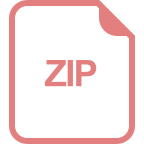
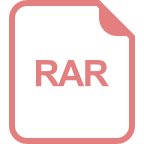
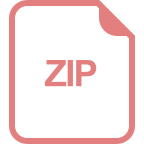
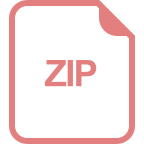
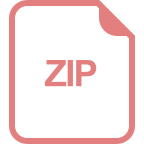
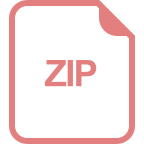
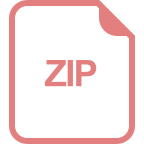
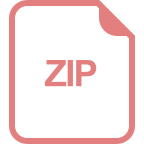



