CH579M:I2C驱动OLED
时间: 2023-10-30 11:24:25 浏览: 201
C知道:你想了解如何驱动 I2C 接口的 OLED 显示屏。使用 I2C 驱动 OLED 很常见,因为它只需要两根线来传输数据和控制信号。首先,你需要确保你的硬件支持 I2C 接口,并连接好你的 OLED 显示屏。
接下来,你需要下载并安装适用于你的微控制器或开发板的相应的 OLED 显示屏库。常见的库包括 Adafruit SSD1306、u8g2 和 Arduino OLED 等。这些库通常提供了一系列函数和方法,方便你进行 OLED 的初始化、写入数据和控制。
在你的代码中,首先你需要初始化 I2C 总线。这通常涉及到设置 I2C 的引脚和速率等参数。然后,你可以使用 OLED 库提供的函数来初始化 OLED 显示屏。
一旦 OLED 初始化完成,你就可以使用库中的函数来写入文本、绘制图形等操作。这些函数将会通过 I2C 总线发送数据给 OLED 显示屏。
当然,在具体实现过程中,你需要查看 OLED 显示屏的文档和库的使用说明,以确保正确地使用库函数和配置 I2C 参数。希望这些信息对你有所帮助!如有任何问题,欢迎继续提问。
相关问题
物联网CH579M驱动OLED实时显示时间的代码
以下是一个基于CH579M芯片和OLED屏幕的物联网应用程序,可以实时显示当前时间:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ch579.h>
#include <ch579usb.h>
#include <ch579i2c.h>
#include <ch579uart.h>
#include <ch579gpio.h>
#include <ch579spi.h>
#include <ch579adc.h>
#include <ch579timer.h>
#include <ch579iic.h>
#include "oled.h"
#define I2C_CLOCK_FREQ 400000
#define OLED_ADDR 0x78
/* OLED I2C接口初始化 */
void oled_i2c_init(void)
{
IICInit(I2C_CLOCK_FREQ);
}
/* OLED初始化 */
void oled_init(void)
{
oled_i2c_init();
OLED_Init();
}
/* OLED屏幕显示时间 */
void oled_show_time(void)
{
/* 获取当前时间 */
RTC_TIME_Type rtcTime;
RTC_GetTime(&rtcTime);
/* 显示时间到OLED屏幕 */
OLED_ShowString(0, 0, "Time: ", 16);
OLED_ShowNum(48, 0, rtcTime.hour, 2, 16);
OLED_ShowChar(64, 0, ':', 16);
OLED_ShowNum(80, 0, rtcTime.minute, 2, 16);
OLED_ShowChar(96, 0, ':', 16);
OLED_ShowNum(112, 0, rtcTime.second, 2, 16);
OLED_Refresh_Gram();
}
int main(void)
{
/* 初始化OLED屏幕 */
oled_init();
/* 初始化RTC时间 */
RTC_TIME_Type rtcTime;
rtcTime.year = 2021;
rtcTime.month = 9;
rtcTime.day = 22;
rtcTime.hour = 0;
rtcTime.minute = 0;
rtcTime.second = 0;
RTC_SetTime(&rtcTime);
/* 循环显示当前时间 */
while (1) {
oled_show_time();
delay_ms(1000);
}
return 0;
}
```
上述代码中,我们使用了CH579M芯片的RTC模块来获取当前时间,并使用OLED屏幕显示当前时间。我们还需要引入一个OLED屏幕的库文件来实现OLED屏幕的初始化和显示功能,这里我们使用了一个名为"oled.h"的头文件,具体实现可以参考如下代码:
```c
#ifndef __OLED_H__
#define __OLED_H__
#include <stdio.h>
#include <string.h>
#include <ch579i2c.h>
void OLED_Init(void);
void OLED_ShowChar(uint8_t x,uint8_t y,uint8_t chr,uint8_t Char_Size);
void OLED_ShowString(uint8_t x,uint8_t y,uint8_t *chr,uint8_t Char_Size);
void OLED_ShowNum(uint8_t x,uint8_t y,uint32_t num,uint8_t len,uint8_t size);
void OLED_ShowChinese(uint8_t x,uint8_t y,uint8_t no);
void OLED_DrawBMP(uint8_t x0, uint8_t y0,uint8_t x1, uint8_t y1,uint8_t BMP[]);
void OLED_Set_Pos(unsigned char x, unsigned char y);
void OLED_Fill(unsigned char fill_Data);
void OLED_CLS(void);
void OLED_ON(void);
void OLED_OFF(void);
void OLED_Refresh_Gram(void);
#endif
```
上述代码中,我们定义了一些函数来实现OLED屏幕的初始化、字符、字符串、数字、图像等显示功能,具体实现可以参考对应函数的定义。
stm32写I2c驱动oled代码
下面是使用STM32的I2C驱动OLED的基本代码示例:
```
#include "stm32f10x.h"
#include "i2c.h"
#define OLED_I2C_ADDR 0x78 // OLED I2C地址
// OLED初始化命令序列
const uint8_t oled_init_cmds[] = {
0xAE, // 关闭显示
0xD5, 0x80, // 设置时钟分频因子,震荡器频率
0xA8, 0x3F, // 设置驱动路数
0xD3, 0x00, // 设置显示偏移
0x40, // 设置显示开始线--0
0x8D, 0x14, // 设置显示模式,开启内部电源
0x20, 0x00, // 设置内存地址模式
0xA1, // 设置SEG方向
0xC8, // 设置COM方向
0xDA, 0x12, // 设置COM硬件引脚配置
0x81, 0xCF, // 设置对比度
0xD9, 0xF1, // 设置预充电周期
0xDB, 0x40, // 设置VCOMH
0xA4, // 全局显示开启
0xA6, // 设置显示方式
0xAF // 开启显示
};
void oled_init(void) {
i2c_start();
i2c_send_byte(OLED_I2C_ADDR << 1);
i2c_send_byte(0x00);
for (uint8_t i = 0; i < sizeof(oled_init_cmds); i++) {
i2c_send_byte(oled_init_cmds[i]);
}
i2c_stop();
}
void oled_set_pos(uint8_t x, uint8_t y) {
i2c_start();
i2c_send_byte(OLED_I2C_ADDR << 1);
i2c_send_byte(0x00);
i2c_send_byte(0xB0 + y);
i2c_send_byte(((x & 0xF0) >> 4) | 0x10);
i2c_send_byte((x & 0x0F) | 0x01);
i2c_stop();
}
void oled_clear(void) {
for (uint8_t i = 0; i < 8; i++) {
oled_set_pos(0, i);
for (uint8_t j = 0; j < 128; j++) {
i2c_start();
i2c_send_byte(OLED_I2C_ADDR << 1);
i2c_send_byte(0x40);
i2c_send_byte(0x00);
i2c_stop();
}
}
}
void oled_write_char(uint8_t x, uint8_t y, char ch) {
uint8_t c = ch - 32;
if (x > 120) {
x = 0;
y++;
}
oled_set_pos(x, y);
for (uint8_t i = 0; i < 6; i++) {
i2c_start();
i2c_send_byte(OLED_I2C_ADDR << 1);
i2c_send_byte(0x40);
i2c_send_byte(Font6x8[c][i]);
i2c_stop();
x++;
}
}
void oled_write_string(uint8_t x, uint8_t y, char *str) {
while (*str != '\0') {
oled_write_char(x, y, *str++);
}
}
int main(void) {
i2c_init();
oled_init();
oled_clear();
oled_write_string(0, 0, "Hello, world!");
while (1) {
}
}
```
以上代码使用了一个Font6x8数组来存储字形数据。你可以使用自己的字形数据替换它。同时,你需要根据你的硬件环境来修改i2c_init()和i2c_send_byte()函数,以确保它们能够正确地工作。
阅读全文
相关推荐
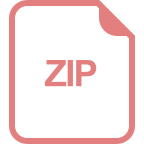
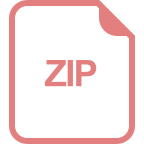
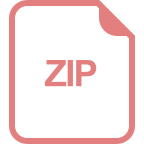
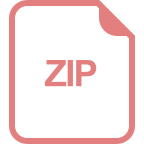
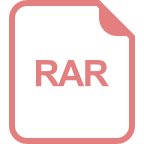
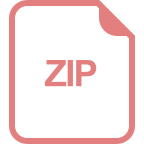
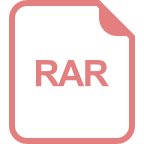
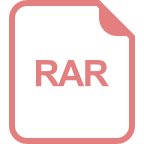
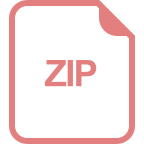
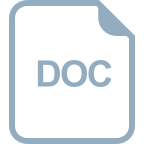


