将顺序表(a1,a2..an)重新排列以a1为界的两部分:a1前面的值均比a1小,a1后面的值均比a1大(这里假设数据 元素的类型具有可比性,不妨设为整型)。 数据结构方法写出代码
时间: 2024-09-18 20:16:33 浏览: 63
可以使用双指针法来解决这个问题,通常这种算法在排序或数组操作中会遇到。以下是使用Python的一个简单示例,假设列表已经包含整数:
```python
def partition_and_sort(lst):
# 初始指针i指向第一个元素,j指向最后一个元素
i = 0
j = len(lst) - 1
while i < j:
# 当左指针所指元素小于a1时,交换并移动左指针
if lst[i] < lst[0]:
lst[i], lst[j] = lst[j], lst[i]
j -= 1
else:
i += 1
return lst
# 示例输入
arr = [4, 6, 2, 9, 1, 5, 8, 7, 3]
sorted_arr = partition_and_sort(arr)
print("Sorted array with a1 as boundary:", sorted_arr)
相关问题
将顺序表(a1,a2,a3,...,an)重新排列为以a1为界的两部分:a1前面的值均比a1小,后面的值均比a1大(数据类型均具有可比性,都设为整型)
这种操作通常被称为“分而治之”的策略,特别是归并排序或类似算法中的思想,但针对给定的顺序表实现起来比较简单。可以采用双指针法,分别从列表两端开始遍历:
1. 初始化两个指针i(左指针)和j(右指针),分别指向列表的首元素a1和最后一个元素an。
2. 当i < j时,执行以下步骤:
a. 检查当前i位置的元素ai是否大于a1,如果不大于,说明左边部分已经满足条件,将i向右移动一位;反之,交换ai和aj(即右边的元素)。
b. i++,j--。
3. 当i >= j时,循环结束。此时列表将分为两部分,a1左边的部分满足条件,右边的部分也是因为已经遍历完毕,所以所有元素也一定大于a1。
以下是伪代码形式:
```python
def partition_and_sort(lst):
if len(lst) <= 1:
return lst
pivot = lst[0]
i = 0
for j in range(1, len(lst)):
if lst[j] < pivot:
lst[i], lst[j] = lst[j], lst[i]
i += 1
lst[0], lst[i] = lst[i], lst[0] # 将pivot放回正确的位置
return lst[:i], lst[i:] # 返回两部分
# 使用示例:
list_to_sort = [5, 2, 9, 1, 7, 6, 8]
sorted_left, sorted_right = partition_and_sort(list_to_sort)
```
写C语言代码完成将顺序表(a1,a2,…,an)重新排列为以a1为界的两部分:a1前面的值均比a1小,a1后面的值均比a1大
你可以使用C语言中的两个指针法来实现这个功能,通常称为“快速排序分区”算法的思想。这里是一个简单的示例,假设我们有一个整数数组`arr[]`:
```c
#include <stdio.h>
// 比较函数,用于确定元素的位置
int compare(int a, int b) {
return a - b;
}
// 分区函数,将数组划分为两部分
void partition(int arr[], int low, int high) {
int pivot = arr[low]; // 选择第一个元素作为基准
int i = low + 1; // 小于基准的元素指针
for (int j = low + 1; j <= high; j++) {
if (compare(arr[j], pivot) < 0) { // 如果当前元素小于基准
i++; // 移动i到下一个位置
swap(&arr[i], &arr[j]); // 交换元素
}
}
swap(&arr[low], &arr[i - 1]); // 将基准放在正确的位置上
}
// 主函数
void rearrange_sequence(int arr[], int n) {
int start = 0; // 前半部分的开始指针
int end = n - 1; // 后半部分的结束指针
while (start < end) {
partition(arr, start, end);
if (arr[start] >= arr[end]) break; // 如果前半部分已经按照要求排列,退出循环
// 交换arr[start] 和 arr[end]
swap(&arr[start], &arr[end]);
start++;
end--;
}
}
// 交换函数
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 测试函数
void print_array(int arr[], int size) {
for (int i = 0; i < size; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {5, 3, 8, 4, 6, 1, 9, 7};
int n = sizeof(arr) / sizeof(arr[0]);
rearrange_sequence(arr, n);
printf("Reordered array: ");
print_array(arr, n);
return 0;
}
```
当你运行这个程序,它会将数组`arr`重新排列,使得`arr[0]`左边的元素都小于它,右边的元素都大于它。
阅读全文
相关推荐
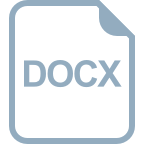
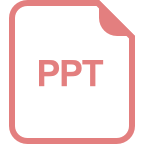
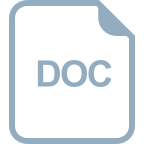










