将顺序表(a1,a2…an)重新排列以a1为界的两部分:a1前面的值均比a1小,a1后面的值均比a1大(这里假设数据 元素的类型具有可比性,不妨设为整型)。 数据结构方法写出代码 c语言
时间: 2024-09-19 12:17:57 浏览: 49
为了将顺序表按照题目描述重新排列,你可以使用双指针法。这是一种常用的算法策略,在这种情况下可以创建两个指针,一个指向列表开始,另一个指向列表结束,然后交换这两个位置的元素直到它们相遇。以下是C语言中的一种实现方法:
```c
#include <stdio.h>
// 假设arr[]是一个整数数组,size是其长度
void partition(int arr[], int size, int *pivotIndex) {
int left = 0; // 左指针
int right = size - 1; // 右指针
while (left < right) {
// 如果左指针处的元素小于 pivot(即 a1)
if (arr[left] < arr[*pivotIndex]) {
// 交换左指针和当前元素的位置
int temp = arr[left];
arr[left] = arr[*pivotIndex];
arr[*pivotIndex] = temp;
// 移动左指针向右
left++;
} else if (arr[right] > arr[*pivotIndex]) { // 如果右指针处的元素大于 pivot
// 交换右指针和当前元素的位置
temp = arr[right];
arr[right] = arr[*pivotIndex];
arr[*pivotIndex] = temp;
// 移动右指针向左
right--;
} else { // 当前元素等于 pivot,不需要交换
left++; // 继续移动左指针
right--; // 继续移动右指针
}
}
*pivotIndex = left; // 更新 pivotIndex 为新找到的 pivot 位置
}
int main() {
int arr[] = {5, 2, 9, 1, 7, 4}; // 示例数组
int size = sizeof(arr) / sizeof(arr[0]);
int pivotIndex = 0; // 初始设定 pivot 为第一个元素
partition(arr, size, &pivotIndex);
printf("Sorted array with pivot at %d:\n", pivotIndex + 1);
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
这个程序首先将 `pivotIndex` 设定为第一个元素的索引,然后通过不断交换左右指针位置,直到它们相遇,保证了数组分区的需求。注意,`main` 函数最后会打印出排序后的数组。
阅读全文
相关推荐
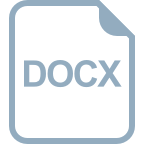
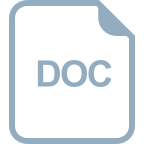
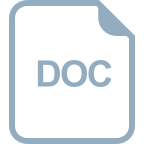












