c语言输入一句英文,进行词频统计
时间: 2024-12-13 13:19:05 浏览: 3
在C语言中进行英文词频统计,通常需要分词、去除停用词(如常见介词、冠词等)、计数以及存储结果。由于C本身并不支持字符串处理库的高级功能,这通常是通过C++的`std::string`、`istringstream`结合一些自定义函数实现。以下是一个简单的示例,假设我们手动进行分割并忽略大小写:
```c++
#include <stdio.h>
#include <ctype.h> // for tolower()
#include <stdlib.h> // for malloc, free
typedef struct {
char *word;
int count;
} WordCount;
void split_words(const char *input, WordCount **counts) {
char *word = strtok(input, " ");
while (word != NULL) {
word = strdup(tolower(word)); // 转化为小写并复制单词
counts = realloc(*counts, (strlen(*counts)+1) * sizeof(WordCount));
(*counts)[strlen(*counts)].word = word;
(*counts)[strlen(*counts)].count = 1;
word = strtok(NULL, " ");
}
}
int main() {
char input[100];
printf("Enter a sentence in English: ");
fgets(input, sizeof(input), stdin);
WordCount *counts = malloc(sizeof(WordCount));
counts->word = NULL; // 初始化计数数组头部
counts->count = 0;
split_words(input, &counts);
for (int i = 0; i <= counts->count; i++) {
printf("%s appears %d times.\n", counts[i].word, counts[i].count);
}
free(counts->word); // 避免内存泄漏
free(counts);
return 0;
}
```
请注意,这个示例非常基础,并未涵盖停用词过滤、错误处理及更高效的计数方法。实际应用中,可以考虑使用第三方库(例如`libstemmer`或`NLTK`),或者编写更复杂的算法来处理。
阅读全文
相关推荐
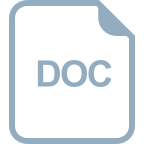
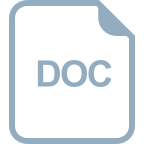
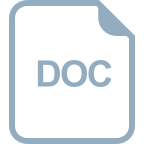
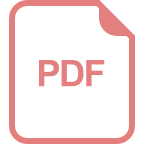
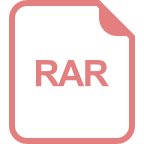
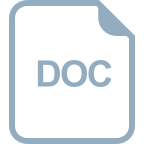

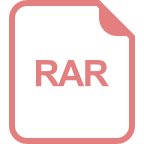
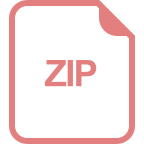
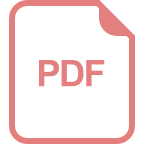
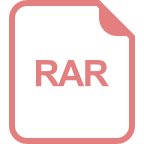
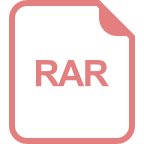
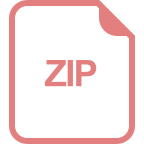
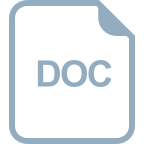
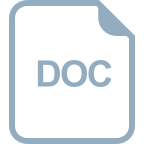
