python多线程 Lost connection to MySQL server during query
时间: 2023-11-01 20:51:10 浏览: 154
你遇到的问题可能是由于在多线程环境下,MySQL连接超时导致的。为了解决这个问题,你可以尝试以下几种方法:
1. 增加MySQL连接超时时间:可以通过设置`connect_timeout`参数来增加连接超时时间,例如:
```python
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
'connect_timeout': 60 # 设置连接超时时间为60秒
}
connection = mysql.connector.connect(**config)
```
2. 使用连接池:使用连接池可以更好地管理和复用数据库连接,避免频繁地连接和关闭数据库。你可以尝试使用`threading.local()`来创建一个线程本地的连接池,确保每个线程都有自己的数据库连接。以下是一个示例:
```python
import mysql.connector
import threading
# 创建线程本地的连接池
pool = threading.local()
def get_connection():
# 如果当前线程没有连接,则创建新的连接
if not hasattr(pool, 'connection'):
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'your_host',
'database': 'your_database',
}
pool.connection = mysql.connector.connect(**config)
return pool.connection
# 在每个线程中使用连接
def query():
conn = get_connection()
cursor = conn.cursor()
cursor.execute('SELECT * FROM your_table')
result = cursor.fetchall()
cursor.close()
# 在多个线程中执行查询操作
threads = []
for _ in range(10):
t = threading.Thread(target=query)
threads.append(t)
t.start()
for t in threads:
t.join()
```
这样,每个线程都会从连接池中获取独立的数据库连接,避免了多线程共享同一个连接的问题。
3. 使用互斥锁:如果你不希望使用连接池,你可以在多线程中使用互斥锁来保证同时只有一个线程在执行数据库查询操作。在每次查询前获取锁,并在查询结束后释放锁。以下是一个示例:
```python
import mysql.connector
import threading
lock = threading.Lock()
def query():
conn = mysql.connector.connect(
user='your_username',
password='your_password',
host='your_host',
database='your_database'
)
cursor = conn.cursor()
# 获取互斥锁
lock.acquire()
cursor.execute('SELECT * FROM your_table')
result = cursor.fetchall()
# 释放互斥锁
lock.release()
cursor.close()
conn.close()
# 在多个线程中执行查询操作
threads = []
for _ in range(10):
t = threading.Thread(target=query)
threads.append(t)
t.start()
for t in threads:
t.join()
```
这样,每次查询前都会获取到互斥锁,确保同一时间只有一个线程在执行数据库查询操作。
你可以根据实际情况选择适合你的方法来解决问题。希望对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐






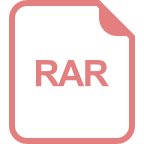





